Want to try it for yourself?
JavaScript security
JavaScript vulnerabilities and best practices explained
Like nearly any programming language, JavaScript is not without its share of potential security exposures. Exploiting JavaScript vulnerabilities can manipulate data, redirect sessions, modify and steal data, and much more. Although JavaScript is typically thought of as a client-side application, JavaScript security issues can create problems on server-side environments as well.
The best defense against common JavaScript security vulnerabilities is to be aware of them and implement the proper controls to reduce exposure.
Javascript Security Vulnerabilities in 2023
The most common JavaScript vulnerabilities include Cross-Site Scripting (XSS), malicious code, Man-in-the-middle attack and exploiting vulnerabilities in the source code of web applications. These can be prevented by scanning your code for vulnerabilities during development and educating your developers about security.
In this article, we’ll take a look at the most common JavaScript vulnerabilities and how to prevent them through popular modern security approaches combined with testing tools (e.g, auditing and code analysis tools, JavaScript vulnerability scanner, etc.)
What Is JavaScript Security?
JavaScript security is related to investigating, preventing, protecting, and resolving security issues in applications where JavaScript is used.
JavaScript itself is a fundamental technology for building web applications and is also very popular for building server-side, desktop, and even mobile applications. It's widespread popularity, however, also makes it a prime target for hackers, looking to target it through various attack vectors. Because JavaScript is used mostly in the front-end, it makes sense to focus first on JavaScript security issues in browsers.
Software vendors have also recognized these JavaScript security issues, reacting with JavaScript security scanning software and a variety of JavaScript security testing tools that make applications more secure and greatly reduce JavaScript security risks. Try out our JavaScript code checker to find vulnerabilities in your code.
What Are the Common JavaScript Vulnerabilities?
Most common JavaScript attack vectors include: executing malicious scripts, stealing a user’s established session data or data from the browser’s localStorage, tricking users into performing unintended actions, and exploiting vulnerabilities in the source code of web applications.
Of course, this list is by no means exhaustive; rather, it is more focused on the front-end aspect of web applications.
8 JavaScript security vulnerabilities
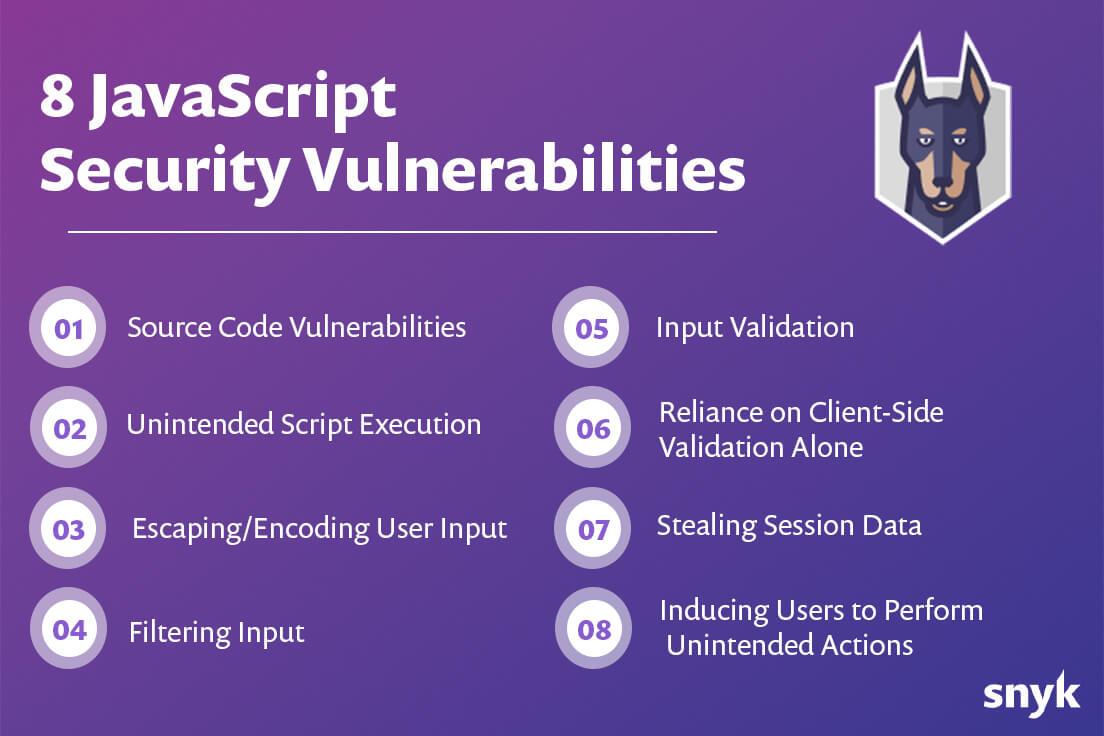
Get started in capture the flag
Learn how to solve capture the flag challenges by watching our virtual 101 workshop on demand.
1. Source Code Vulnerabilities
Frequently, source code vulnerabilities may be combined with other—even a number of—JavaScript security holes. Unfortunately in such cases, using a single JavaScript obfuscation cannot prevent or hide these types of vulnerabilities. Because JavaScript is an interpreted, not a compiled, language, it would be virtually impossible to protect application code from being examined by potential hackers with this method. Nonetheless, obfuscation is still a good practice, as it slows down the hackers in their reverse-engineering attempts.
Another cause of security holes in the source code is the widespread use of public packages and libraries. NPM, a lead player in the JavaScript ecosystem, offers more than a million packages in its registry. While the sheer variety offered is certainly an advantage, this also means there are potentially a huge number of hidden vulnerabilities in these packages that are installed in web application projects.
Moreover, developers often install packages even for the simplest tasks, thus expanding their project dependencies. This of course can lead to security issues and have other far-reaching consequences.
While monitoring and addressing all potential application dependency vulnerabilities can be time-consuming and labor-intensive, auditing tools can help to automate and thus accelerate the process.
A multi-pronged approach for preventing JavaScript security issues in source code should include:
Improving awareness of best practices among developers
Proper auditing of application code to detect potential vulnerabilities
Writing unit tests not only to ensure that code behaves as expected, but also that it executes securely
Implementing tools to scan applications dynamically and identify JavaScript security issues in third-party packages and libraries
2. Unintended Script Execution
The majority of unintended script execution attacks involve cross-site scripting (XSS). A particular concern related to JavaScript is the way it interacts with the Document Object Model (DOM) on a web page, allowing scripts to be embedded and executed on client computers across the web. And so, while several different types of XSS attacks exist, what they all have in common is that they cause untrusted script to appear and run in the user's browser.
One of the most basic XSS attack scenarios is often seen on forum websites in which users can see each other’s messages on the page. If HTML or JavaScript is not properly encoded when they are part of of a message, this could enable unscrupulous users to post a script into a forum.
Posting such a script would make every end user a victim unintentionally facilitating the attack by simply running the application, with the malicious code appearing to be part of the web page.
To prevent XSS attacks, developers should apply sanitization—a combination of escaping, filtering, and validating string data—when handling user input and output from the server.
3. Escaping/Encoding User Input
XSS attacks rely on supplying data that contains certain special characters that are used in the underlying HTML, JavaScript, or CSS of a web page. When the browser is rendering the web page and encounters these characters, it sees them as part of the code of the web page rather than a value to be displayed. This is what allows the attacker to break out of a text field and supply additional browser-side code that gets executed.
To prevent this, any time browser-supplied data will be returned in a response (whether immediately reflected or retrieved from a database), these special characters should be replaced with escape codes for those characters.
For instance, the < and > characters used to delimit HTML entities can be replaced with < and >, which tells the browser to display those characters rather than interpret them as HTML entities. If the browser-supplied data is being returned in a JavaScript context, non-alphanumeric characters should be escaped using xNN where NN is the hexadecimal ASCII value of the character.
4. Filtering Input
In some cases, it might be preferable to simply remove dangerous characters from the data received as input. This can provide some degree of protection but should not be relied on alone for protection from data manipulation. There are various techniques attackers can use to evade such filters.
5. Input Validation
Whenever possible, browser-supplied input should be validated to ensure it only contains expected characters. For instance, phone number fields should only be allowed to contain numbers and perhaps a dash or parentheses characters. Input containing characters outside the expected set should be immediately rejected. These filters should be set up to look for acceptable characters and reject everything else.
6. Reliance on Client-Side Validation Alone
While all of the methods discussed above are good and work well in browsers, hackers may use special tools to send data directly to the server, thus avoiding client-side validations. This would allow entry of potentially malicious or unverified data to the server. Without additional server-side validation, stored data could be corrupted or replaced with erroneous data.
The recommended best practice for preventing such scenarios is to implement both client and server-side validation. This approach reduces the risk of bad data, while still providing the validation functions on the client that improve results for the end user.
Server-only validation can be a nuisance to the user, since it may require filling out online forms multiple times before all validations are passed. JavaScript validation should be used to inform the user immediately of issues with their input, while server validation ensures only expected data makes its way to the application.
7. Stealing Session Data
Client-side browser script can be very powerful in that it has access to all the content returned by a web application to the browser. This includes cookies that could potentially contain sensitive data, including user session IDs. In fact, a common exploit of XSS attacks is to send the user’s session ID tokens to the attacker so they can hijack the session.
To prevent this, most browsers now support the Http-Only attribute on cookies. When the server sets a cookie on the browser, setting the Http-Only attribute tells the browser not to allow access to the cookie from the DOM. This prevents client-side script-based attacks from accessing the sensitive data stored in those cookies.
Local and session storage browser data can also be stolen in the same way, though it cannot be secured through DOM access. It is therefore best to avoid storing sensitive information, such as tokens, in browser storage unless necessary due to specific features of the web application architecture.
8. Inducing Users to Perform Unintended Actions
Cross-site request forgery (CSRF) attacks attempt to trick a browser into executing malicious requests on the websites the user is already logged in to, even if the site is not actually opened at that time. If sessions on the target site are cookie-based, requests to that site can be automatically enriched with authorization cookies.
Hackers can also implement their own web pages and have them perform malicious requests to other sites in the background when the user opens it. They can also use social media, forums, and other platforms to post malicious links or other content that forces browsers to make unnoticed calls to other sites using the user's session cookies.
The general technique for avoiding this vulnerability is implementing tokenization of client-server communication in which an additional token is introduced that is not stored in cookies. Tokens should be generated for each form on the website upon session establishment and should be sent together with each request while the user is present on the website.
How to Deal with JavaScript Security Issues?
Protecting applications and servers from JavaScript vulnerabilities can be managed through the adoption of JavaScript security best practices and the use of sophisticated scanning tools.
In the world of web development, software engineers must constantly keep on top of new JavaScript security risks that arise. Not only is it important to conduct functionality tests on applications; using JavaScript security testing tools on a regular basis is also key for preventing vulnerabilities. Last, following some simple and common best practices will definitely increase the durability of your applications.
The following JavaScript security best practices can reduce this risk.
Avoid**eval()**: Don’t utilize this command in code, since it simply executes passed argument if it is a JavaScript expression. This means if the hacker succeeds in manipulating input value, he or she will be able to run any script she wants. Instead, opt for alternative options that are more secure.
Encrypt:Use HTTPS/SSL to encrypt data exchanged between the client and the server.
Set secure cookies:To ensure SSL/HTTPS is in use, set your cookies as “secure,” which limits the use of your application’s cookies to only secure web pages.
Set API access keys:Assign individual tokens for each end user. If these tokens don’t match up, access can be denied or revoked.
Use safe methods of DOM manipulation:Methods such as innerHTML are powerful and potentially dangerous, as they don’t limit or escape/encode the values that are passed to them. Using a method like innerText instead provides inherent escaping of potentially hazardous content. This is particularly useful in preventing DOM-based XSS attacks.
Identifying potential JavaScript security problems is an essential first step toward preventing vulnerabilities in application development. Test your code with an open-source vulnerability scanner now.
Snyk for JavaScript security
From your first line of code to your last npm dependency, Snyk keeps your JavaScript applications secure right from your IDE, CLI, and Git workflows.
Up Next
Storytime by Tanya Janca - Secure Coding Libraries
Listen to Tanya share her best practices for creating templates for secure code.
Keep reading