5 tips for securing PHP Laravel

DeveloperSteve Coochin
April 21, 2022
0 mins readDeveloper-centric Laravel is often described as “the PHP framework for web artisans.” It’s one of the most used frameworks within the PHP ecosystem, with an extensive community and a wide array of sub-branches dedicated to application development of all kinds.
With version 1.0 launched in 2011, Laravel currently powers the websites of global companies like 9GAG, BBC, and Pfizer. The main project, having grown so much over the years, is broken down into a few different branch components, which are used extensively for websites and platforms alike:
Laravel/core - This is the main focus point for much of the community and the recommended starting place for anyone looking to get started with Laravel. This project contains everything you need to get a website up and running quickly, with easy-to-follow tutorials and documentation. PHP Composer has a rolling average of over 16000 installs over 24 hours for Laravel/core.
Laravel/framework - This provides the core Laravel functionality as a framework that is used by many other projects as a dependency. It has been installed over 183 million times since its PHP composer listing in 2013, with an average of over 86000 daily installs. It is used as a dependency by over a million open source platforms and products.
Laravel Sails - This is built around a lightweight CLI specifically designed to work with Laravel’s default dockerized development environment. Sails is designed for building applications with Laravel using a containerized approach for speed and scalability.
Getting started with PHP Laravel
Getting up and running with Laravel is quick and easy, especially using PHP’s package manager, PHP Composer. Starting a new project is as simple as running composer create-project Laravel/Laravel example-app
at the command line. From there, you can use the server built into the Laravel framework by switching to the newly created directory with cd example-app
, and then running php artisan serve
. This starts a local server, which by default will be running on 127.0.0.1:8000.
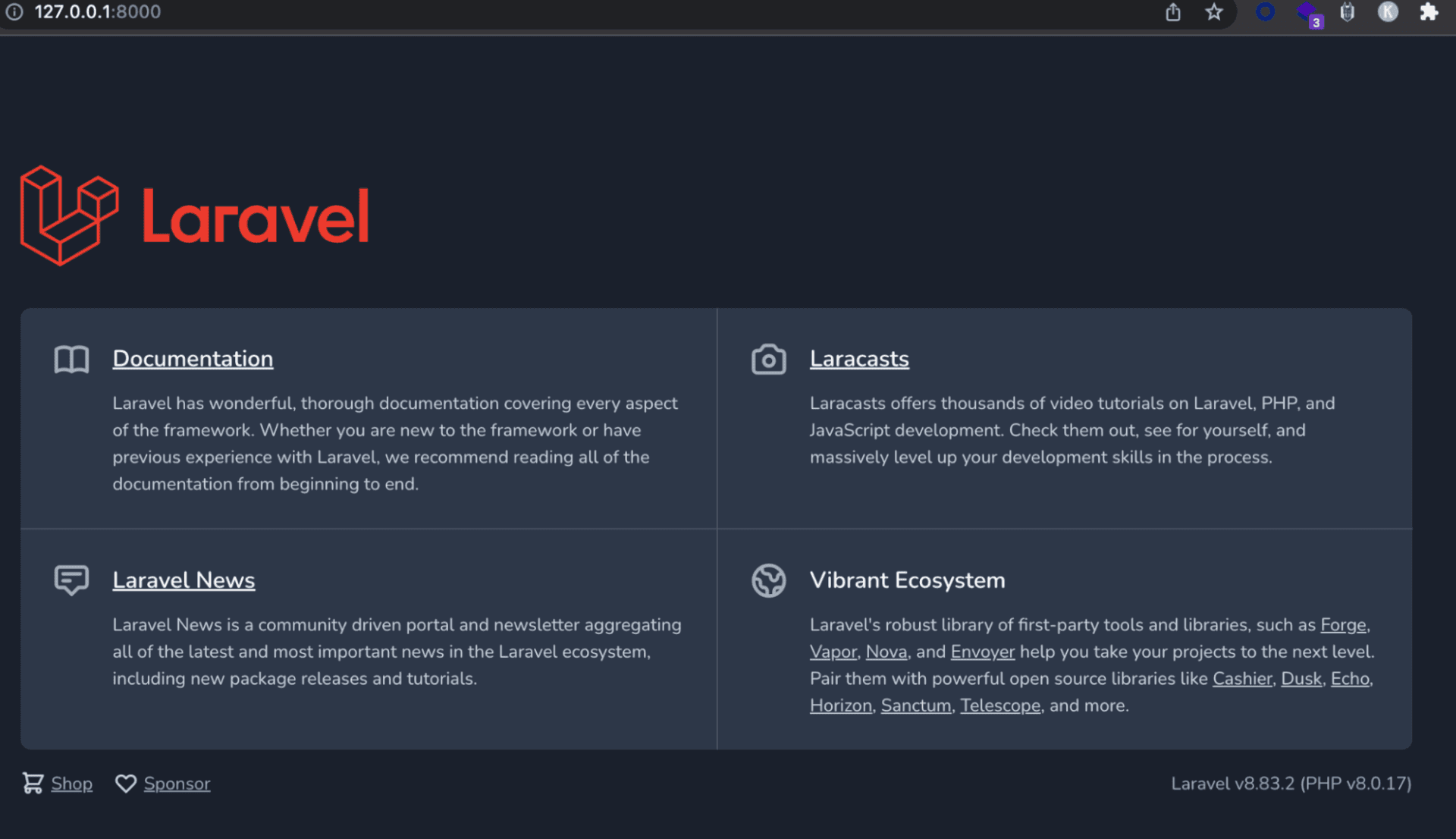
One of the things I love about Laravel is that its developer-centric approach is so well-crafted: tools that developers use frequently are built in. Laravel can automatically create a new git repo from the command line using the --git flag
; you can even specify a branch using the --branch="main"
flag.
Top 5 ways to secure Laravel
Let's look at some ways to secure your Laravel installs and help keep users safe.
1. Framework configuration
Setting up correctly — and early — for the different stages of application development is vital to any project. With Laravel, you can set development and production configurations using the .env file. This file should never be publicly accessible, as it contains configuration information for the application.
In production, make sure that debug mode is turned off. You can do this by configuring APP_DEBUG
to false
. While debug mode is useful during the development phase, turning it off in production ensures that no unwanted errors, paths, or critical system information are accessible to public eyes.
It's also important to make sure that an application key has been generated for your Laravel installation. This is used for symmetric encryption with cookies, session-signed URLs, and password reset tokens. To set it up, use key:generate
normally via the artisan commands (or php artisan key:generate
, depending on your Laravel setup).
2. Preventing SQL injection
One of Laravel's biggest advantages is the use of the Eloquent ORM within its core framework. This uses a PDO binding to protect from SQL injection when interacting with the connected database.
An ORM is software that facilitates handling database records by representing data as objects, working as a layer of abstraction on top of the database engine used to store an application's data.
As an additional layer on top of Eloquent, especially when dealing with user input, core PHP can provide more sanitization. You can never have too much sanitization (except when it affects page load and performance). You can learn more about this in our post on the top 5 ways to prevent SQL injection with PHP.
3. Cookies and session management
Protecting user sessions and cookies is a great way to ensure that end user data is kept as safe and secure as possible. There are a few things to keep in mind when dealing with this inside Laravel.
Enable HttpOnly
as part of your application’s Laravel session – you can do this in the config/session.php
file. This will set in place an additional layer of security and will stop session cookies from being available within Javascript. Set the low session idle timeout value so that inactivity will automatically log out the user.
The cookie domain configuration is also located in the config.session.php
file. It should be set to Null
unless using subdomains. Setting the cookie domain attribute will enforce same origin
, so only the base domain can set the cookie. It's also a good practice to set the SameSite
cookie attribute to lax
or strict
to restrict the cookies to first-party or same-site.
The most important configuration option in the config/session.php
file is HTTPS only
. Enabling it protects against MITM (man in the middle) attacks, and enforces that only HTTPS secure URLs are allowed.
4. Securely handle uploads
Always validate the file type using MimeTypes:
1$request->validate([
2 'photo' => 'file|size:100|mimes:jpg,bmp,png'
3]);
Additionally:
Don’t rely on users to input paths or filenames. Always use hash strings or system-generated names.
Avoid XML and zips if possible, or handle them in a safe way.
Use
basename()
to strip out unwanted traversals.
5. Use SAST and SCA tools
These tools help keep your code and open source package use as secure as possible by running scans on the code and package manifests. More importantly, they allow you to set up ongoing scanning so you can be alerted if a particular version of a package contains a detected vulnerability.
Read our guide to using SAST paired with SCA for better security coverage for your project.
Software composition analysis (SCA)
SCA tools — like Snyk Open Source — are especially handy when you’re using open source package management software like PHP Composer. An SCA tool can scan the composer manifest to check particular open source package versions for potential known vulnerabilities.
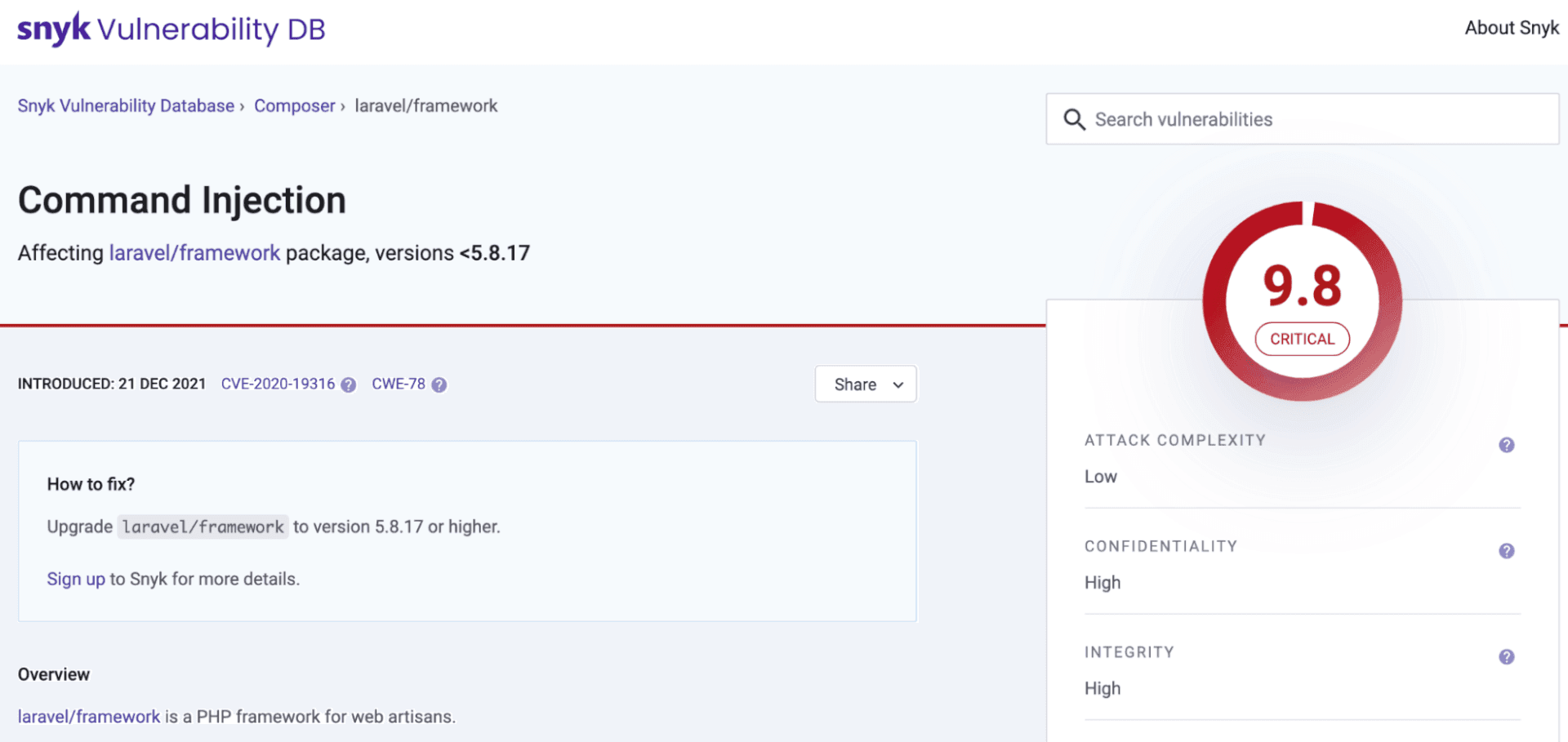
Note that a good SCA tool will continuously scan your projects, open source libraries, and dependencies for vulnerabilities. Year after year, we see an increase in emerging vulnerable paths within ecosystems. Early identification can alert you to newly identified threats, like the recent PHP dompdf vulnerability.
Static application security testing (SAST)
SAST tools — like Snyk Code — can help with the identification of potential vulnerabilities being surfaced in a codebase. This enables you to investigate the identified section of code.
The Snyk Code scan for PHP is particularly helpful because it not only highlights areas of concern, but also helps you trace back through functions, objects, and frameworks to further investigate.
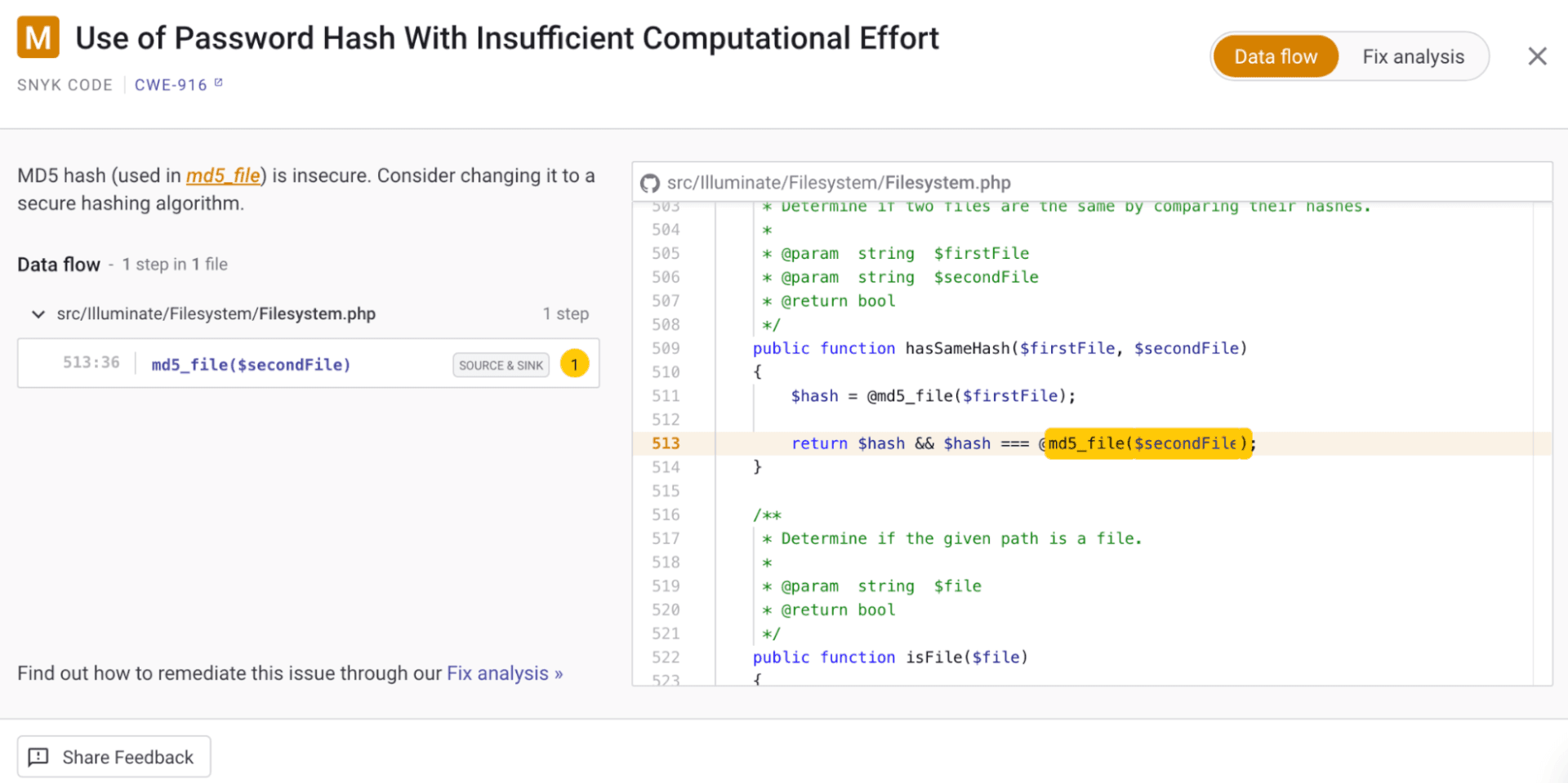
Using real-world examples of the vulnerability in question, Snyk Code also provides potential remediation steps.
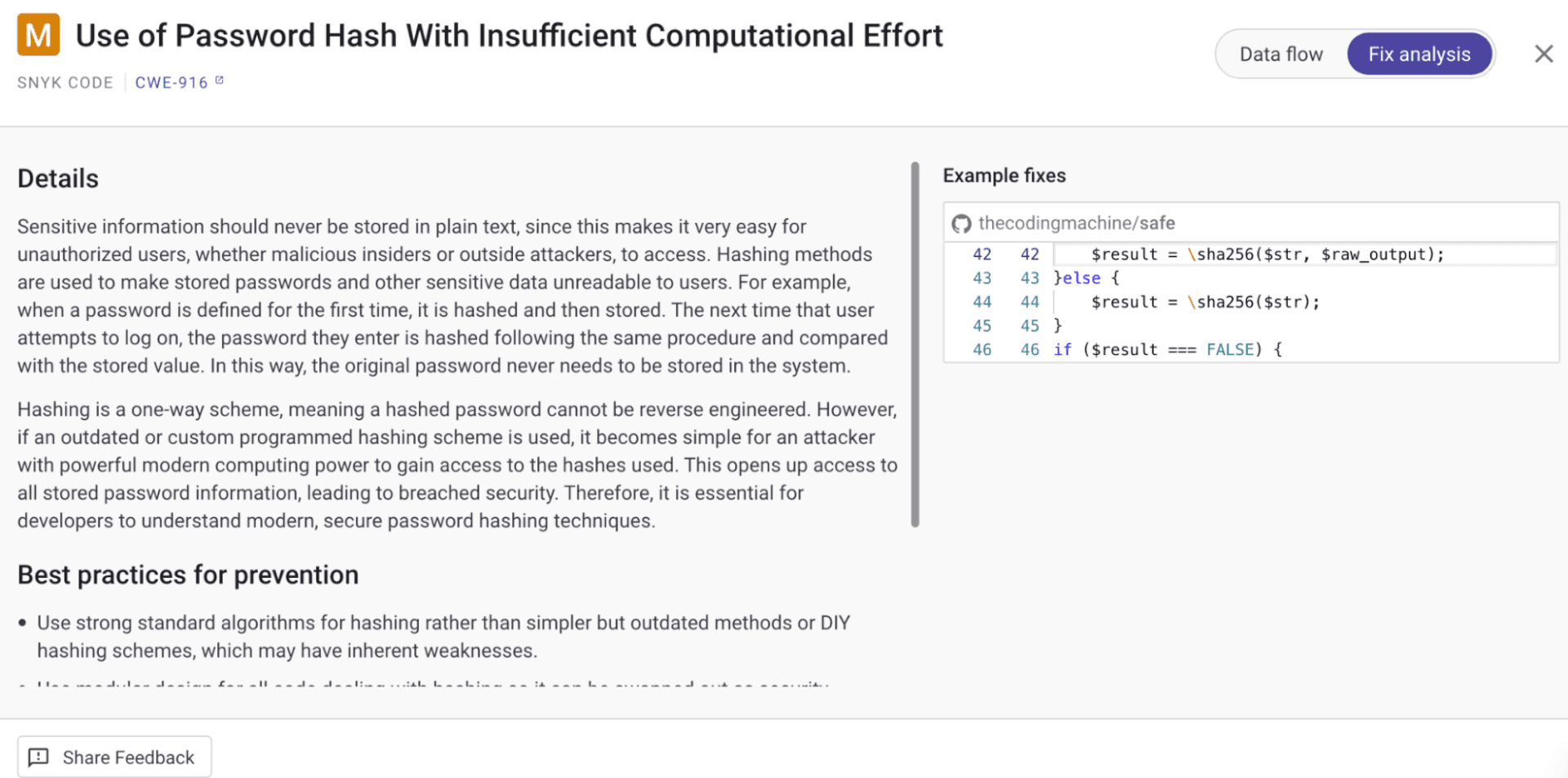
Scan your Laravel projects quicker than you can say “PHP artisan”
Scanning your Laravel projects with Snyk is quick and easy. Get started by creating a free Snyk account and following the login for your preferred platform. After creating an account, you have a few different ways to start scanning projects.
Connect your repository
This is by far my favorite (and the easiest) way to get ongoing alerts for emerging vulnerabilities. From your Snyk dashboard, click Add Project at the top right and select your repository of choice. If it's not in the quick menu, click Other and select your desired platform from the integrations page.
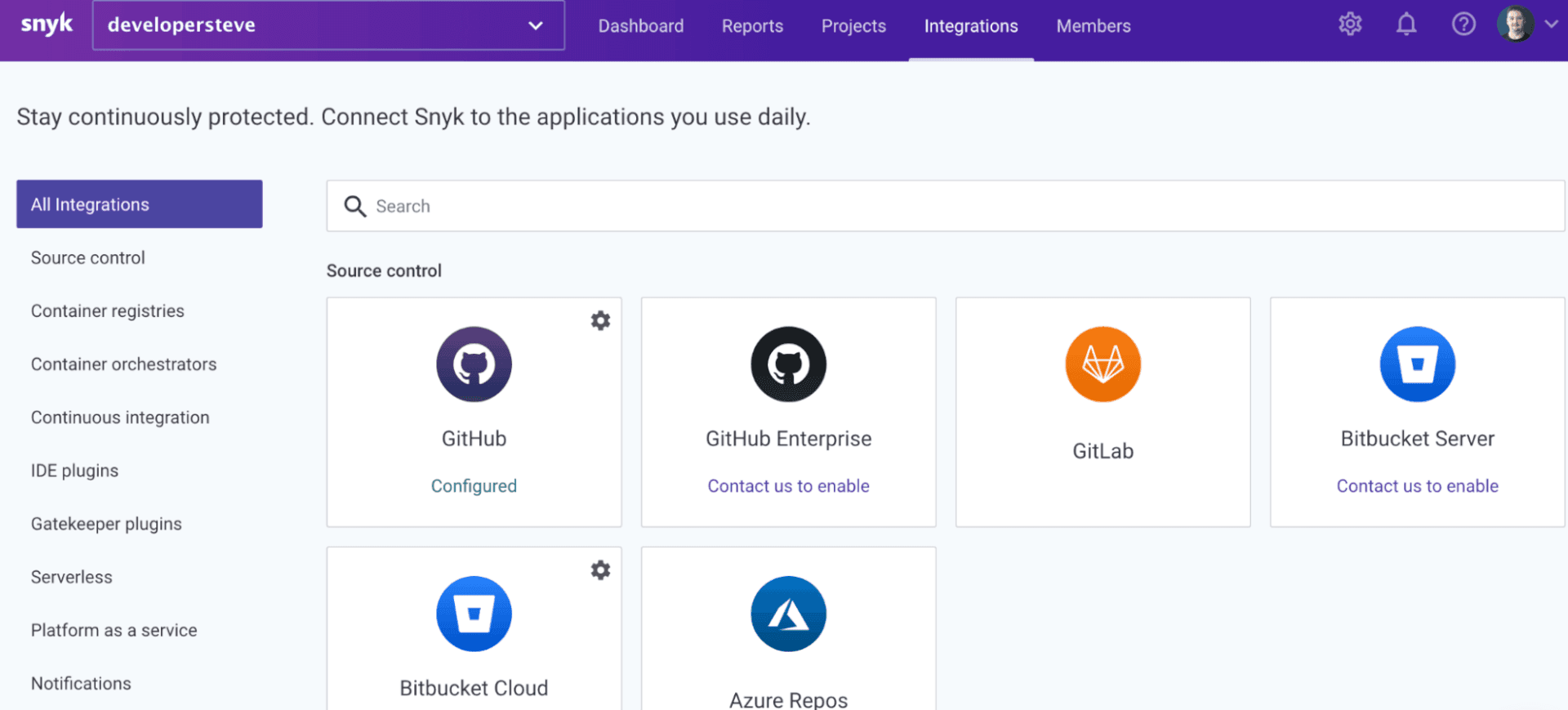
After following the steps to connect your account, you’re ready to connect your projects repository from the configuration page. Check the box for the repository type and click Add selected repository.
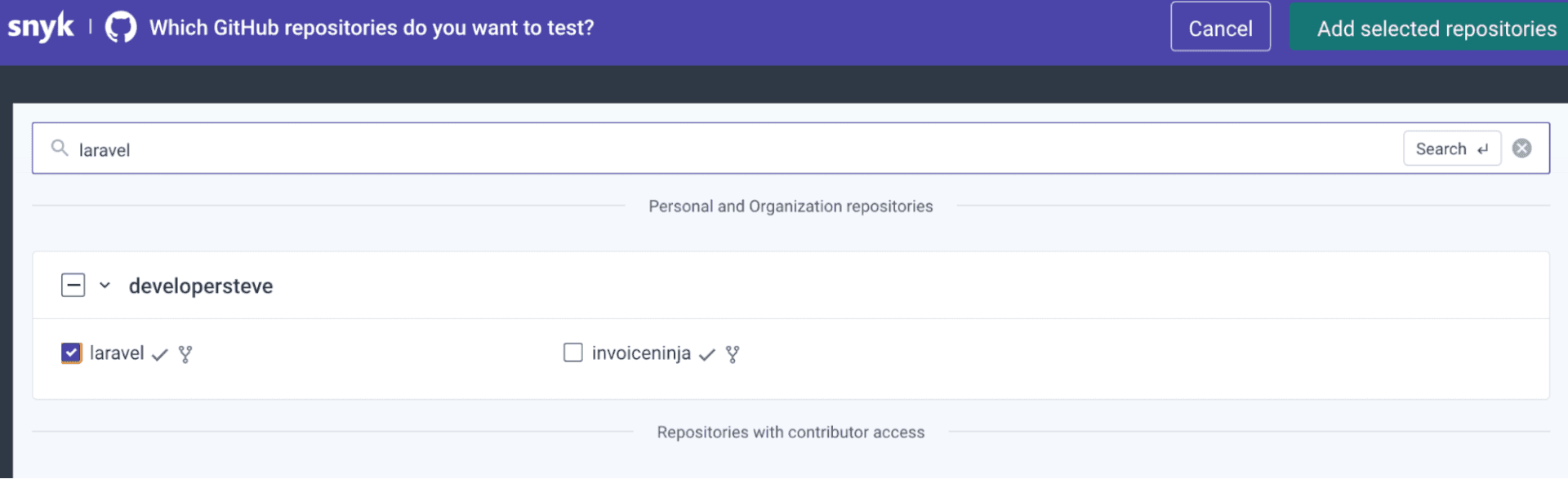
Install the Snyk CLI
The Snyk CLI provides a few ways to build SCA and SAST tooling directly into your command line by simply running a few lines of code. You can do this either via npm or Yarn, or by using standalone executables or operating system-specific installs.
After the Snyk CLI is installed, verify the installation by running snyk –version
to make sure everything is working correctly. Next, connect the Snyk CLI to your Snyk account by running snyk auth
on the command line. This should open your default browser to the authentication page.
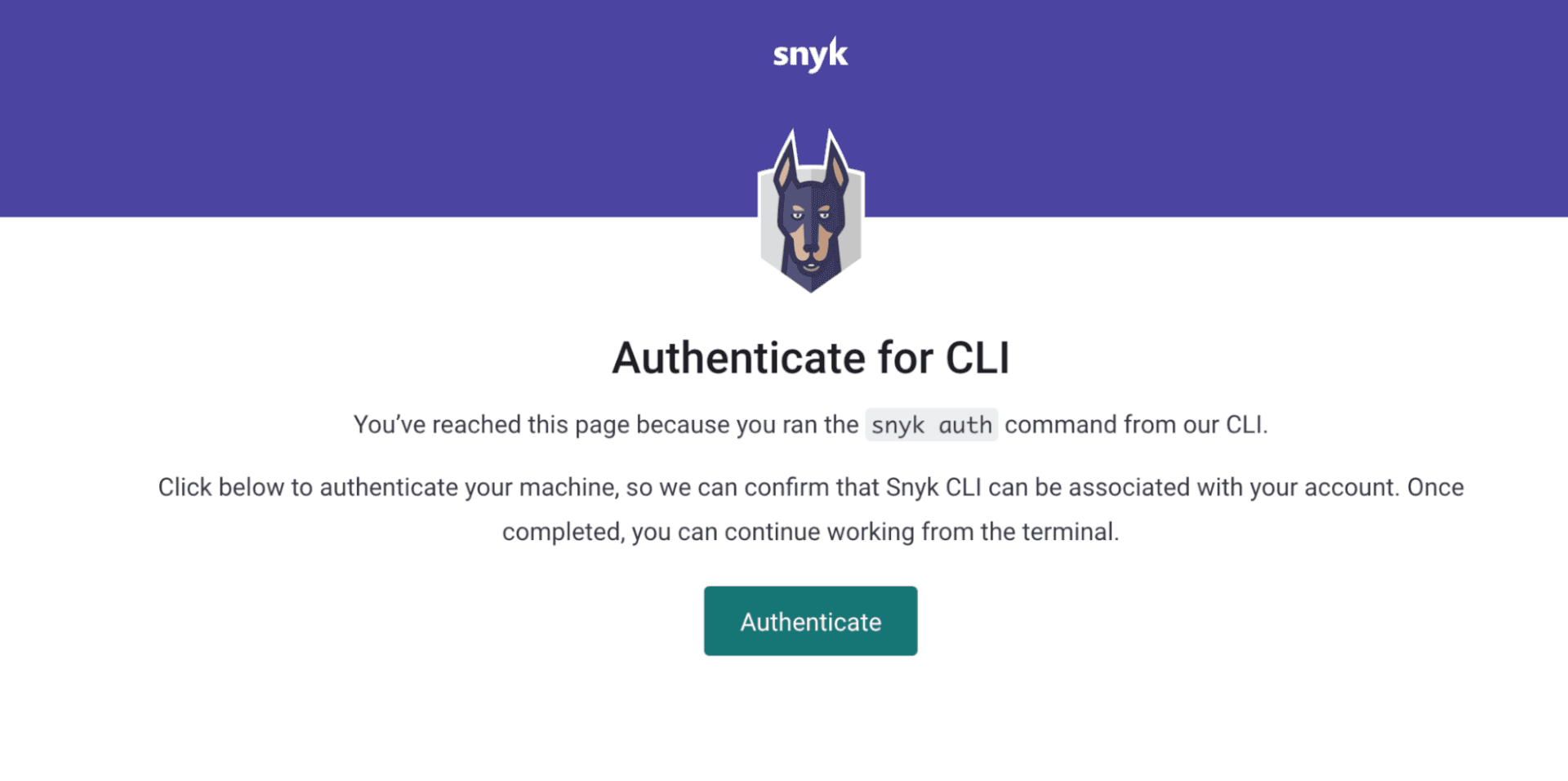
Click Authenticate
, and you’re ready to scan a project using my favorite CLI command: snyk test
.
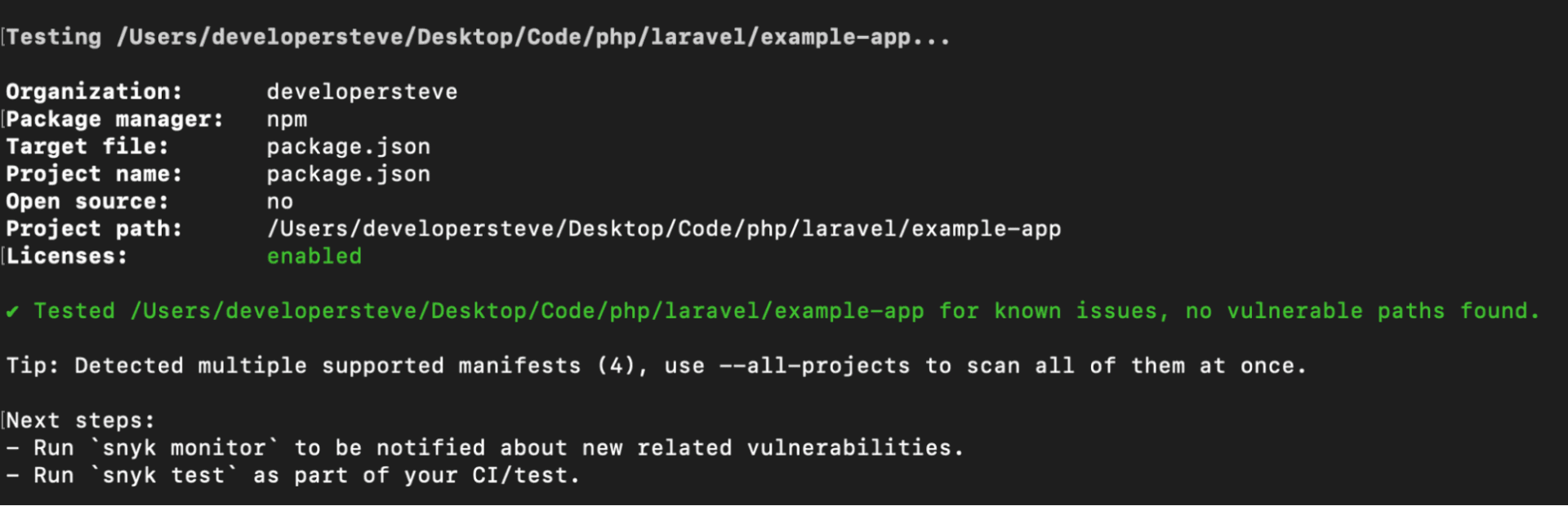
IDE integrations
These scanning tools can also be integrated into your IDE, which does real-time checks and scanning as you build an application. For example, the Snyk VSCode integration includes real-time package and dependency scans. See how to get started and how the Visual Studio code integration works in real time.
Remember to give back to open source projects
Always remember to contribute where you can to any open source projects, particularly if you use them regularly. The Laravel community has grown substantially over the years as functionality and adoption have increased, which has helped make the project what it is today.
Get started in capture the flag
Learn how to solve capture the flag challenges by watching our virtual 101 workshop on demand.