How to write your first unit test in JavaScript
Raphael Mun
October 28, 2022
0 mins readTesting code is the first step to making it secure. One of the best ways to do this is to use unit tests, ensuring that each of the smaller functions within an app behave as they should — especially when the app receives edge-case or invalid inputs, or inputs that are potentially harmful.
Why should we unit test?
When it comes to unit testing, there are many different approaches. Some primary purposes of unit tests are:
Verifying functionality: Unit tests ensure that code does the right thing and doesn’t do anything it’s not supposed to — which is where most bugs occur.
Preventing code regression: When we find a bug, adding a unit test to check the scenario keeps code changes from re-introducing the bug in the future.
Documenting code: With the right unit tests, a complete set of tests and results provides the specifications for how an app is supposed to work.
Securing your app: Unit tests can check for exploitable vulnerabilities (such as those that enable malicious SQL injection).
Scoping and writing unit tests
Using a unit test framework enables us to quickly write and automate our tests and integrate them into our development and deployment processes. These frameworks often support testing in both front and back-end JavaScript code.
Below are some general guidelines to help you write performance unit tests and testable code.
Keep unit tests short and simple
Don’t overload your unit tests. The test should only be a couple of lines of code that check short, functional chunks of your application.
Consider both positive and negative test cases
Writing a test to confirm that a function executes properly is helpful. However, it’s far more effective to write a broader set of tests that check whether a function fails properly if misused or in edge cases. These negative tests can be even more valuable because they help anticipate unexpected scenarios, such as when a function should throw an exception or how it should handle receiving malformed data.
Break apart long and complex functions
Large functions that contain a lot of logic are difficult to test; including too many operations prevents testing each variable effectively. If a function is too complex, split it into smaller functions for individual testing.
Avoid network and database connections
Unit tests should be quick and lightweight, but functions that make network calls or connect to other apps or processes take a long time to execute. This makes it challenging to run many operations simultaneously and can create more fragile code. You can use mocking in unit tests to enable simulated network or database calls — this lets you test the rest of the function. You can include the real network and database connections during a different test process called integration testing.
How to code unit tests
Now that we’ve reviewed some unit testing best practices, you’re ready to write your first unit test in JavaScript.
This tutorial uses the Mocha Framework — one of the most popular for unit testing. Each test framework is slightly different, but they’re similar enough that learning the basic concepts will enable you to switch between them easily.
To follow this demonstration, ensure you have Node.js installed on your computer.
Creating a new project
Start by opening a terminal window or command prompt to a new project folder. Then, create a new Node.js project inside it by typing npm init -y
.
This creates a package.json
file inside the folder, enabling you to install Mocha as a development dependency using npm install -D mocha
.
Next, open the package.json
file in your code editor and replace the placeholder test script with mocha
:
1 "scripts": {
2 "test": "mocha"
3 },
Implementing a class
Next, code a simple traffic light system to unit test.
Inside your project directory, create a file called traffic.js
and add the following code for a TrafficLight
class:
1class TrafficLight {
2 constructor() {
3 this.lightIndex = 0;
4 }
5
6 static get colors() {
7 return [ "green", "yellow", "red" ];
8 }
9 get light() {
10 return TrafficLight.colors[ this.lightIndex ];
11 }
12 next() {
13 this.lightIndex++;
14 // This is intentionally wrong!
15 if( this.lightIndex > TrafficLight.colors.length ) {
16 this.lightIndex = 0;
17 }
18 }
19}
20
21module.exports = TrafficLight;
This class consists of four parts:
TrafficLight.colors
: a constant property for the traffic light colors.lightIndex
: a variable tracking the index of the current traffic light color.light
: a class property that returns the current traffic light color as a string.next()
: a function that changes the traffic light to the next light color.
Configuring and adding our first unit test
Now it’s time to add some unit tests around the code.
Create a folder in the project directory called test
. This is where Mocha will check for unit tests by default. Then, add a file named traffic.test.js
inside the new test folder.
Next, import the TrafficLight
class at the top of the file:
1const TrafficLight = require( "../traffic" );
We’ll also use the assert
module for tests, so require it in your code:
1const assert = require( "assert" );
With Mocha, we can group unit tests into sets using the describe()
function. So we can set a top-level group for this class as follows:
1describe( "TrafficLight", function () {
2});
Then, we’ll create some unit tests to validate the traffic colors in their own sub-group, called colors
inside the TrafficLight
set:
1describe( "TrafficLight", function () {
2 describe( "colors", function () {
3 });
4});
For this first unit test, we can verify that colors
only has three states: green, yellow, and red. The test is defined using an it()
function inside the describe()
group, so write the test like this:
1describe( "TrafficLight", function () {
2 describe( "colors", function () {
3 it( "has 3 states", function () {
4 const traffic = new TrafficLight();
5 assert.equal( 3, TrafficLight.colors.length );
6 });
7 });
8});
Now let’s run the unit test and see if it passes.
Run npm test
in your terminal window, and if everything is correct, Mocha prints out the results of your unit test run.
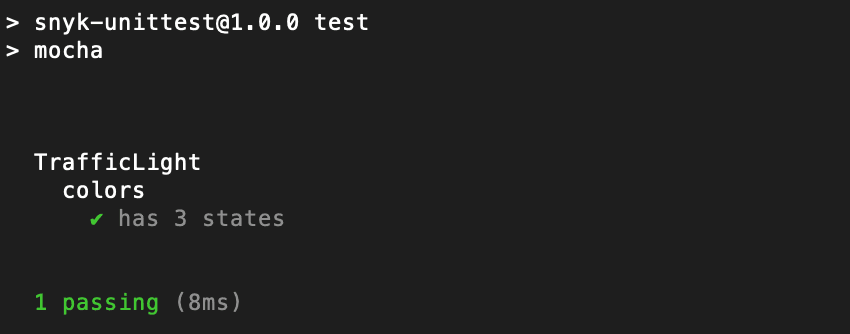
Adding more unit tests
Our project is now ready to run unit tests, so we can add more tests to ensure our code is working properly.
First, add a unit test to the colors
group to validate that the traffic light colors are correct and in order. Here’s one way to implement this test:
1it( "colors are in order", function () {
2 const expectedLightOrder = [ "green", "yellow", "red" ];
3 const traffic = new TrafficLight();
4 for( let i = 0; i < expectedLightOrder.length; i++ ) {
5 assert.equal( expectedLightOrder[ i ], TrafficLight.colors[ i ] );
6 }
7});
Second, test the next()
function to see if it changes the traffic lights correctly. Create a new sub-group and add two unit tests: one to check that the lights change in the correct order, and another to check that the light loops after red
back to green
.
Write these unit tests as follows:
1describe( "next()", function () {
2 it( "changes lights in order", function () {
3 const traffic = new TrafficLight();
4 for( let i = 0; i < TrafficLight.colors.length; i++ )
5 assert.equal( traffic.light, TrafficLight.colors[ i ] );
6 traffic.next();
7 }
8 });
9 it( "loops back to green", function () {
10 const traffic = new TrafficLight();
11 // Change the light 3x to go from green -> yellow -> red -> green
12 for( let i = 0; i < 3; i++ ) {
13 traffic.next();
14 }
15 assert.equal( traffic.light, TrafficLight.colors[ 0 ] );
16 });
17});
Now, when we rerun the tests, we’ll see that one of the tests fails. This is because there’s a bug in the TrafficLight
class.
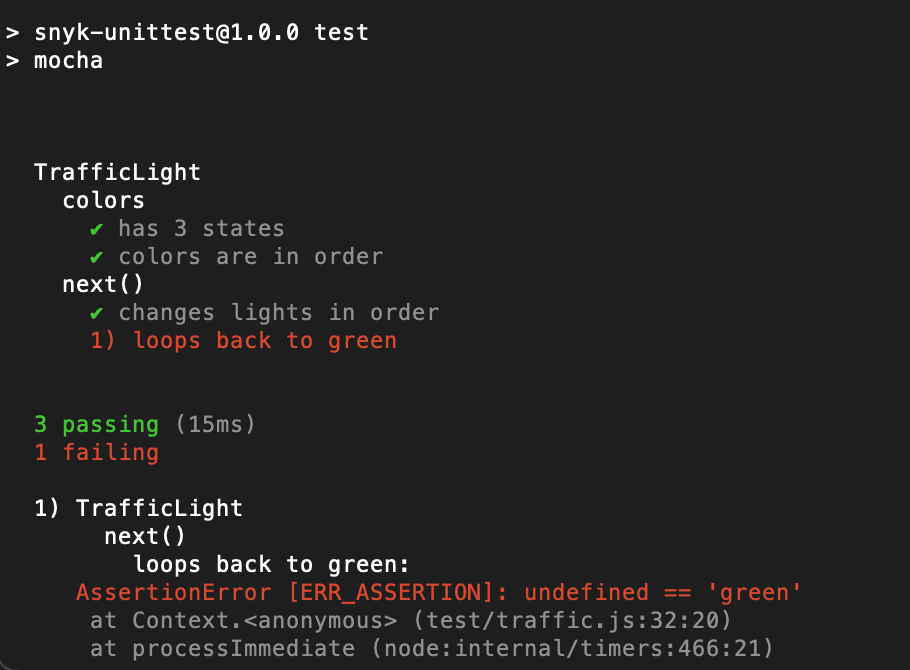
Fixing the bug
Open the TrafficLight
class code again and locate the comment inside the next()
function that reads // This is intentionally wrong!
From our unit tests, we know that this function isn’t correctly looping back to green
. We can see that the code currently checks for when the lightIndex
value surpasses the number of traffic light colors, but the index begins at 0
. Instead, we must loop back as soon as the value reaches the exact number of colors. Let’s update the code to reset the lightIndex
value back to 0
when it is equal to the length of the list of traffic light colors:
1// This is intentionally wrong!
2if( this.lightIndex === TrafficLight.colors.length ) {
3 this.lightIndex = 0;
4}
Now all of your unit tests should pass. And the best part is that even if the TrafficLight
class becomes refactored or modified heavily, our unit tests will catch this bug before it reaches the user.
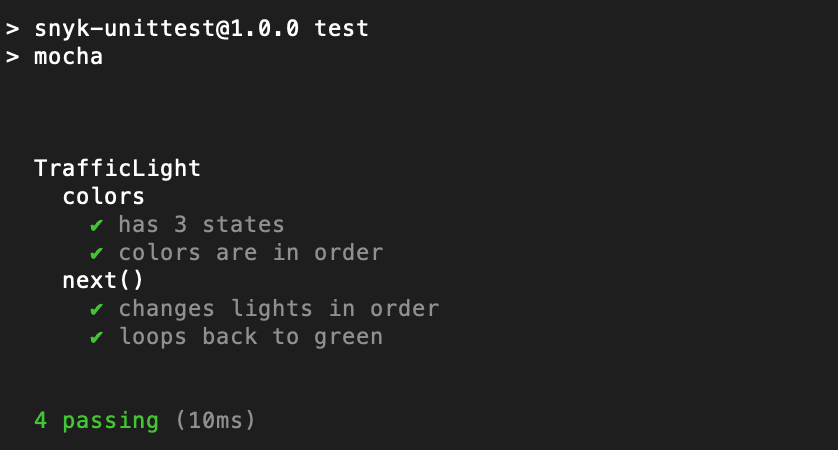
Wrapping up
Unit tests are easy to set up and an effective tool for software development. They help eliminate bugs early and prevent them from returning. This keeps projects more manageable and easy to maintain, even as they become larger and more complex — especially among bigger development teams. Automated tests like these also enable developers to refactor and optimize their code without worrying about whether the new code is behaving correctly.
Unit tests are a key part of your development process and are crucial to help you build better and more secure JavaScript apps.
Happy testing!
Get started in capture the flag
Learn how to solve capture the flag challenges by watching our virtual 101 workshop on demand.