Command injection in Python: examples and prevention

December 21, 2023
0 mins readDespite Python's reputation for simplicity and versatility, ensuring the security of Python programs can be challenging if you or other team members neglect security best practices during development.
Additionally, you’ll likely use libraries or other open source projects while building a Python application. However, these resources can introduce additional security issues that leave your program vulnerable to exploits such as command injection. This critical security flaw can spell disaster for your Python applications. Command injection exposes your applications to unauthorized command execution, potentially leading to data breaches, system compromise, and other malicious activities.
In this article, you’ll learn all about command injection, including how this vulnerability can manifest in your programs. You'll also learn about common security best practices to safeguard your Python apps from command injection attacks.
What is command injection?
Command injection occurs when an attacker can execute arbitrary system commands by injecting them into a vulnerable program. The vulnerability typically arises when an application passes unsafe user data (ex: forms, cookies, and HTTP headers) to a system shell.
Several scenarios can lead to command injection vulnerabilities, including passing unsanitized user input to system commands. Attackers can inject malicious arguments when an application directly passes user input into a command line. An example of this is the command injection vulnerability found in certain versions of MLflow (machine learning lifecycle platform). This vulnerability stems from insufficient sanity checks on the predict()
method in the backend.py
file.
Additionally, applications might dynamically construct command strings based on various parameters, including user input. These can be exploited if the construction process lacks proper checks and validation.
Unfortunately, it is easy to misunderstand the implications of system commands. Misusing or misconfiguring system commands can easily introduce command injection vulnerabilities. For instance, affected versions of PaddlePaddle, the parallel distributed deep learning platform, are vulnerable to comman injection in fs.py
via the os.system
method.
The consequences of command injection are severe. Attackers can gain unauthorized access, exfiltrate sensitive data, or corrupt the system. Breaches like this can result in financial losses, reputational damage, and legal repercussions for organizations, as well as untold damage to users whose sensitive information may be stolen.
Understanding and fixing command injection in Python
Command injection remains one of the most severe threats to application security. Ensuring the safety of Python applications from command injection requires an in-depth understanding of these vulnerabilities and proactive measures to counteract potential exploits.
Common vulnerabilities leading to command injection
The following section outlines some common vulnerabilities that lead to command injection in Python.
Exploiting user-controlled inputs
User-controlled inputs are data or values directly provided by the user, such as form data, URL parameters, or inputs via the command line. When an application processes these inputs without proper validation and uses them directly to execute system commands, it becomes vulnerable to command injection attacks.
For instance, take a look at this Python example:
# Vulnerability: Directly using user-controlled input to execute a command.
command = input("Enter a command to execute: ")
os.system(command)
This code prompts the user to provide a command through the input method. This user-controlled input is then directly passed to the os.system
method for execution without undergoing any form of validation or checking, meaning the user inputs are instantly processed and executed by the system's command interpreter:
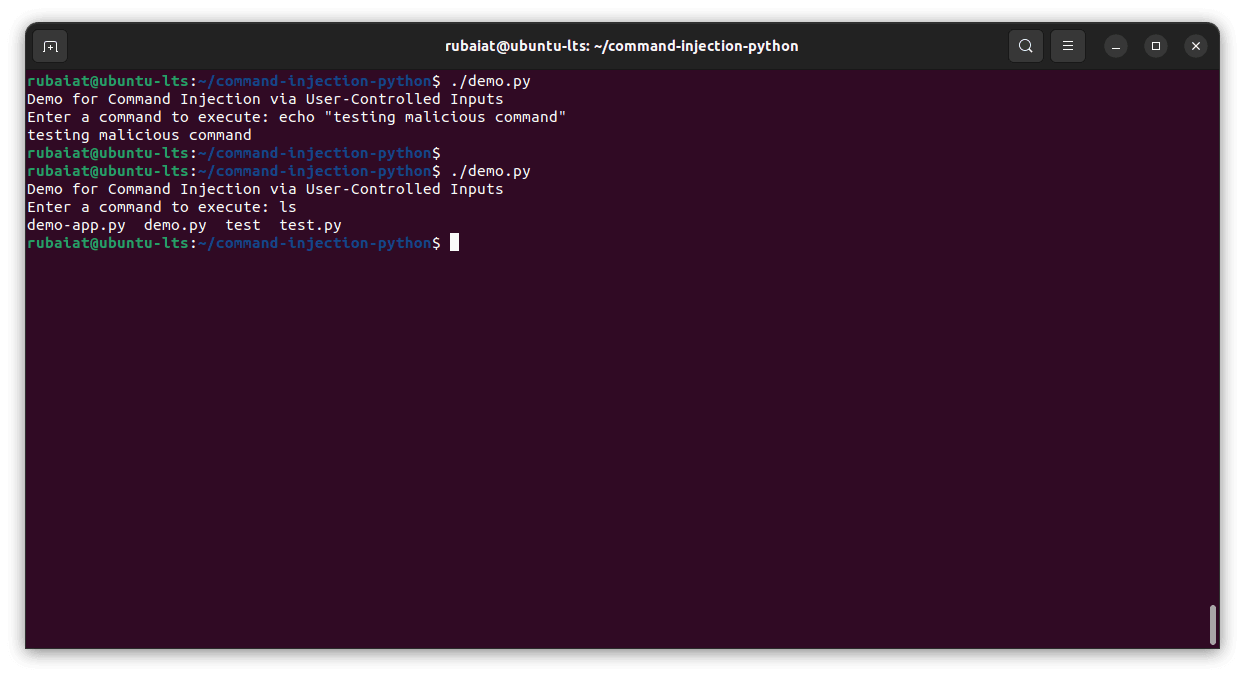
In this simple example, basic shell commands are used to test code. However, attackers will likely use dangerous commands that cause permission escalation, data loss, and other unwanted scenarios.
Insecure use of system commands and the subprocess module
System commands and the subprocess module in Python allow for the execution of shell commands, providing robust ways to interact with the underlying operating system. However, when not used securely, they can introduce command injection vulnerabilities, especially when combined with user-controlled inputs.
To illustrate, take a look at this vulnerability:
# Vulnerability: Using subprocess with shell=True and unsanitized user input.
command = input("Enter a command for subprocess: ")
subprocess.run(command, shell=True)
Here, user input is directly executed using the subprocess.run
method with shell=True
enabled. The shell=True
argument means that the command is executed by the system shell, which allows for command chaining and other potentially malicious operations:
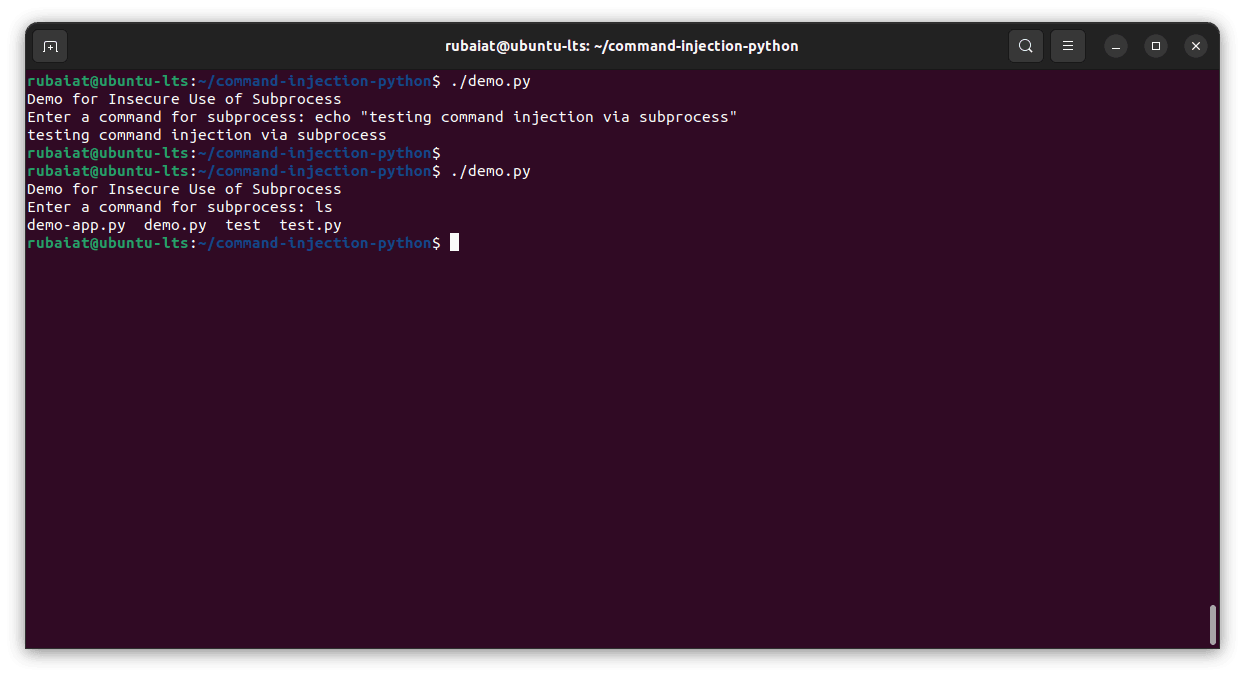
By not validating or sanitizing the user input, the script exposes itself to command injection attacks, essentially handing over the power of the system shell to potential attackers.
Risks associated with dynamic command construction
It's not uncommon for Python programs to build and execute system commands dynamically, especially when there's a need to incorporate variable data, like user inputs. However, when this dynamic construction lacks proper security measures, it paves the way for command injection vulnerabilities.
Take a look at the following Python snippet:
# Vulnerability: Dynamically constructing commands without sanity checks.
user_input = input("Enter a parameter: ")
command = f"echo Printing {user_input}"
os.system(command)
This code prompts the user for a parameter and then constructs a command string that incorporates this input directly. This command is intended to print the user's input. However, because there's no validation or sanitization of the user input before it's embedded into the command, it's vulnerable to command injection:
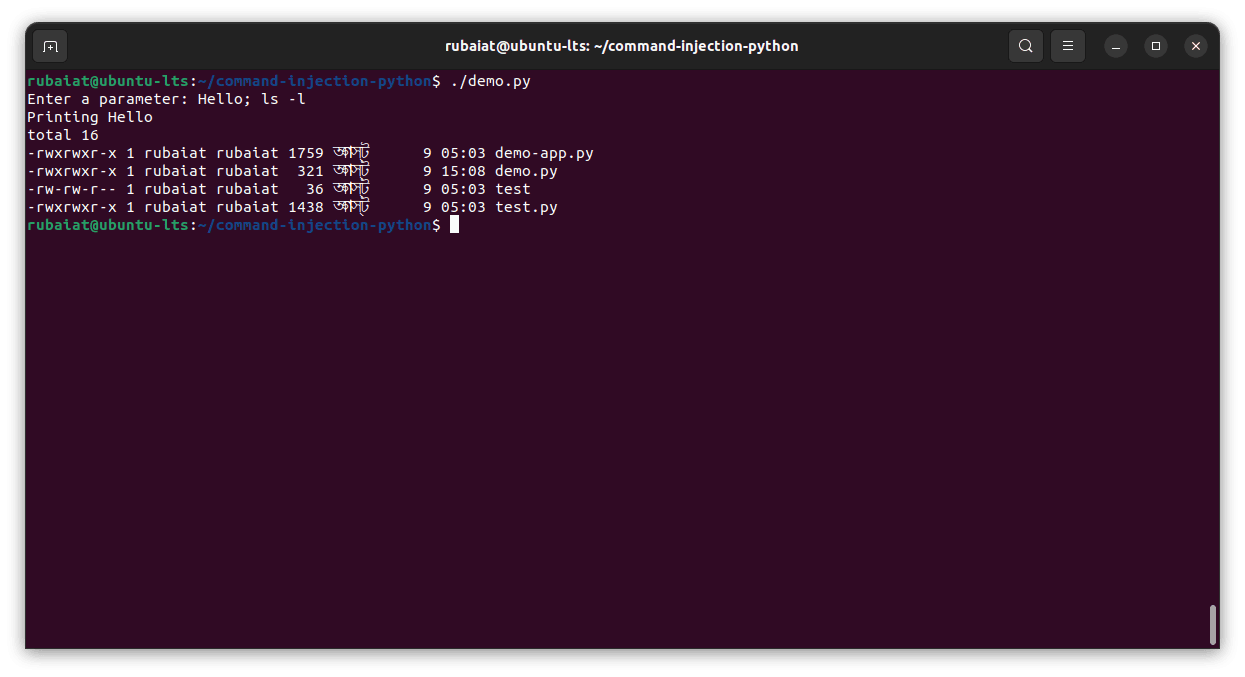
An attacker can exploit this vulnerability by entering a payload that includes command separators or control operators. For instance, a user could input Hello; ls -l
, which would first print "Hello"
and then list the contents of the current directory due to the semicolon command separator. It's a simple example, but attackers can input more malicious commands, such as rm -rf /
or cat /etc/shadow
.
Insecure use of eval()
Python's eval()
function dynamically evaluates a string as a Python expression and returns the result. While it's a powerful tool, it's also a double-edged sword. Consider the following Python snippet:
# Vulnerability: Insecure use of the eval() method.
user_input = input("Enter a Python expression to evaluate: ")
try:
result = eval(user_input)
print(f"Result:\n{result}")
except Exception as e:
print(f"Error:\n{e}")
Using eval()
in this code is dangerous because it doesn't just evaluate mathematical or simple Python expressions, but any Python code. When combined with Python's capability to interact with the underlying system using modules like os
.
For example, an attacker can input the following:
__import__('os').popen('ls').read()
The example input above imports the os
module and then runs the ls
command on Unix-based systems, listing the directory contents. This is a simple demonstration, but malicious actors can input more harmful commands to manipulate files, exfiltrate data, or even gain unauthorized access to the system:
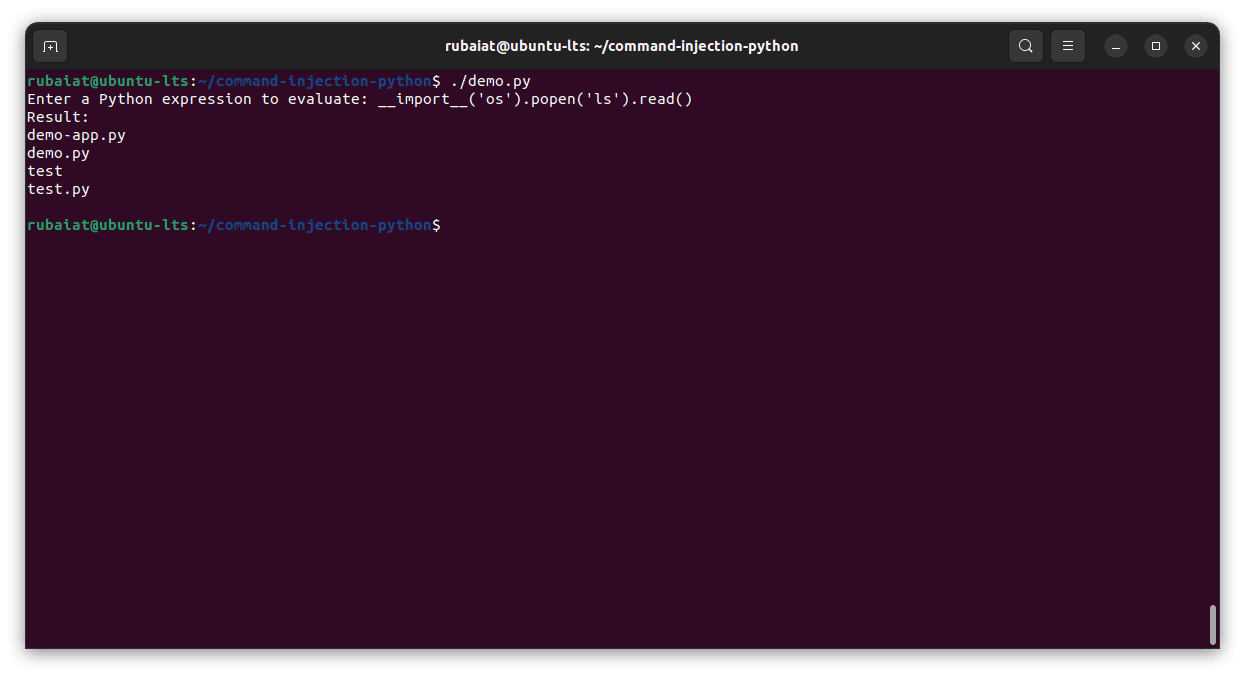
Mitigating command injection vulnerabilities
Given the potential negative impact of command injection on your Python applications, it's crucial to address this issue effectively. Fortunately, you can proactively mitigate numerous command injection vulnerabilities early in the software development lifecycle (SDLC) by incorporating the following guidelines.
Implement proper input validation and sanitization
Input from users or external sources should never be trusted. Always validate input against expected patterns and sanitize it:
import re
def sanitize_input(user_input):
# A simple example: Allow only alphanumeric characters
return re.sub(r'[^a-zA-Z0-9]', '', user_input)
Note that the example above is only stripping non-alphanumeric characters from the input. While this will remove the possibility for attackers to chain commands together, in a real application you’ll likely need much stricter sanitization, for example, allowing only certain known responses, etc.
Use parameterized queries and prepared statements
A parameterized query is a way to structure a command where you first define the command and its structure and then provide the parameters that should be inserted into the command separately. In this way, the command and data are never concatenated as strings. Instead, they remain distinct, and the system treats the Fdata only as data, not executable code.
A prepared statement takes this concept a step further. The command's structure is first prepared, then you merely send the parameters to fill in the placeholders. For example, Python's subprocess module allows for command execution in a manner that separates the command from its arguments, similar to parameterized queries:
import subprocess
command = "ls"
directory = input("Which directory to list? ")
subprocess.run([command, directory])
Use shell=True with caution
When using Python's subprocess module, avoid the shell=True
argument, especially with user inputs. When you execute a command with shell=True
, the system shell interprets any string you pass. If this string contains user input or is constructed from multiple sources without proper sanitization, an attacker can inject malicious commands:
command = input("Enter the directory to list: ")
subprocess.run(f"ls {command}", shell=True)
This is a recipe for disaster, making way for unauthorized system access, data theft, malware installation, privilege escalation, and arbitrary command execution. Try to avoid using shell=True
, or if you must, sanitize the input first.
Avoid passing user input into os methods
Passing user input directly in os
methods, such as os.spawn
or similar, leaves your Python application vulnerable to command injection. That's why you should use alternative Python APIs if they exist for your use case. If you need an os
method, try using it without user input. If you can't do without user input, sanitize everything and use context-aware encoding techniques.
Limit privileges and implement strong access controls
Always run your application with the least privileges necessary. If an attacker does exploit a vulnerability, this limits the damage they can cause. Additionally, consider isolating your application using tools or techniques such as chroot, containers, or user namespaces.
Secure coding conventions in Python
The following sections discuss some secure coding conventions that, when implemented, can help protect your Python applications from command injection vulnerabilities.
Properly validate and sanitize inputs
Input validation and sanitization are integral to safeguarding your Python applications from command injection. By rigorously validating and properly sanitizing user inputs, you effectively reduce the attack surface, prevent unauthorized command execution, and enhance the overall security of your software.
Take a look at the following code:
def get_username():
username = input("Enter your username: ")
if len(username) < 3 or not username.isalnum():
raise ValueError("Invalid username!")
return username
This function ensures that the input is of a specified length and only contains alphanumeric digits before processing. Note that in a real-world application, you’ll likely need stricter validation rules than shown in this simple example.
Use the subprocess module and similar functions carefully
The subprocess module can spawn new processes, connect to their input, output, and error pipes, and obtain their return codes. This means it's essential to use this module securely.
Avoid using shell=True
with the subprocess module unless necessary, as this can execute commands in a shell, leading to command injection attacks:
# Avoid
subprocess.run("ls -l", shell=True)
# Instead Use
subprocess.run(["ls", "-l"])
Safely handle user input and external commands
Never concatenate or interpolate user inputs directly into your command strings. Always use argument lists:
user_input = input("Enter a directory name: ")
# Avoid
subprocess.run(f"ls {user_input}", shell=True)
# Prefer
subprocess.run(["ls", user_input])
Use SAST and SCA tools to find and fix security issues
Static application security testing (SAST) and software composition analysis (SCA) tools can help you analyze both the code you’re writing, as well as the open source components your software uses, to identify vulnerabilities early in the development lifecycle.
The Snyk platform makes finding and fixing security issues as easy as possible due to our hybrid AI approach, which finds and fixes vulnerabilities in your code, providing you with real-time feedback on potential security risks and remediation advice. Using SAST and SCA tools like Snyk (which has a free-forever plan) helps you continuously monitor for security issues in your codebase and correct them early, before they hit production. This means we can help to prevent the following:
Exposure to malicious packages that can compromise your system or data
Supply chain attacks, where a trusted component gets compromised and affects all software that relies on it
Outdated dependencies that may have unpatched vulnerabilities
Overall, incorporating SAST and SCA tools like Snyk into your development process ensures that you're not just building functional software, but also safeguarding against the ever-evolving landscape of cyber threats.
Best practices for secure python development
In this section, you'll explore high-level coding habits and workflows that you can implement to promote secure Python development across teams.
Perform regular code reviews and security audits
Regular code reviews, including thorough examinations of input validation, authentication mechanisms, and sensitive data handling, can help identify potential vulnerabilities early in the development lifecycle. By involving team members specializing in security, you can catch issues that may be missed during initial development, such as insecure coding practices, misconfiguration of settings, and unsanitized inputs.
Conducting periodic security audits is crucial to ensure that your codebase remains robust against evolving threats. You can use tools like OpenVAS, ZAP, and Metasploit to test your program for vulnerabilities.
Keep software and libraries up-to-date
Outdated software and libraries often contain known security vulnerabilities. You should continually update your Python interpreter, frameworks, and third-party libraries to their latest versions. Use package managers like pip and config files like requirements.txt
to manage and update dependencies efficiently.
Leverage security tools and frameworks
You can utilize security tools and frameworks designed for Python to automate vulnerability detection. Tools like Snyk can help identify code-level issues, security misconfigurations, and vulnerabilities in third-party packages.
Find and fix Python vulnerabilities
Secure your applications with Snyk’s vulnerability scanning and fix advice.
No credit card required.
Or Sign up with Azure AD Docker ID Bitbucket
By using Snyk, you agree to abide by our policies, including our Terms of Service and Privacy Policy.
Encourage a security-focused mindset
Fostering a culture of security awareness among developers is vital. Try to provide security training, workshops, and resources to empower developers on security matters. When developers prioritize security from the start, the overall software quality improves, reducing the risk of vulnerabilities.
A great resource for learning more about building security-focused company cultures is the Secure Developer Podcast, which contains numerous interviews with security leaders at companies and dives deep into the way they build their development programs.
IDE extensions for real-time vulnerability detection are valuable tools for identifying vulnerable code as you write it. These extensions integrate directly into your development environment and highlight potential security issues in real-time:
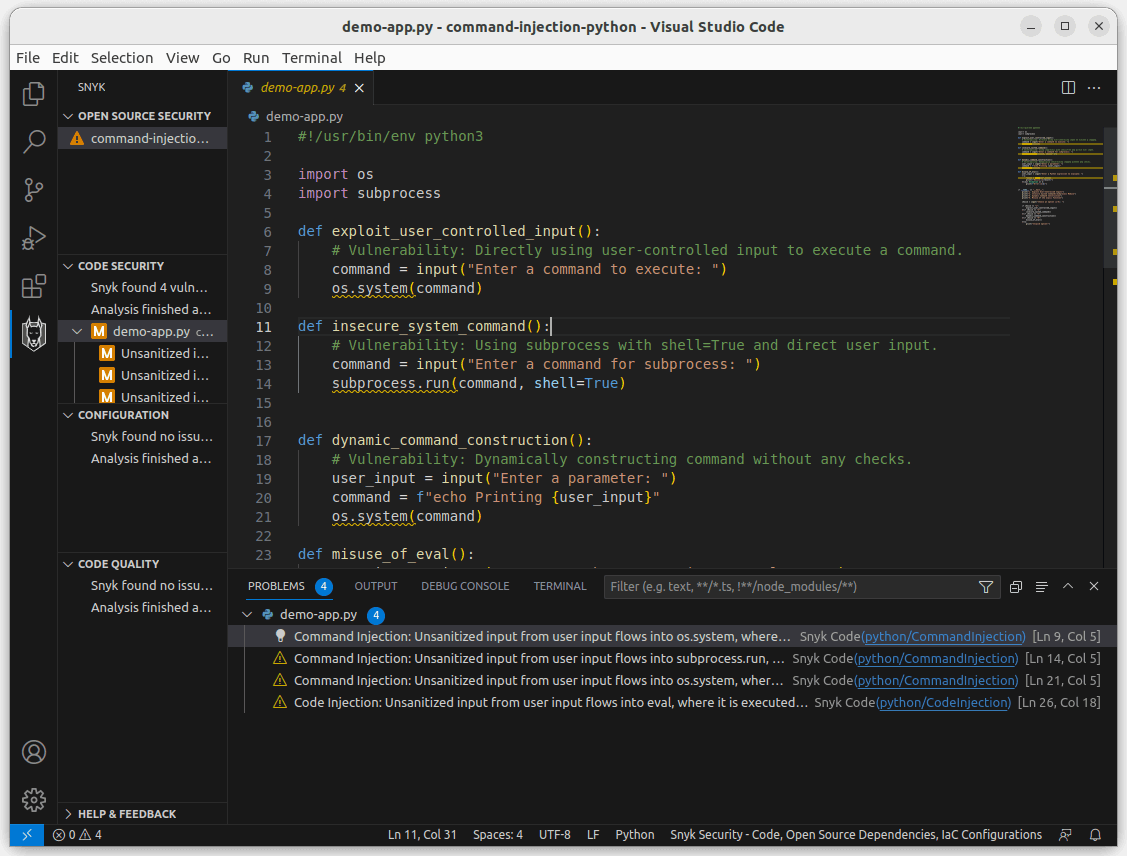
When you catch vulnerabilities during development, you save time and effort that will otherwise be spent later on in the development cycle, when the time and effort it takes to remediate them will be far more costly.
Conclusion
Command injection is a serious problem that affects many Python applications. This guide taught you how this vulnerability can manifest and its associated risks. By recognizing vulnerable code patterns, you can gain valuable insights into potential threats and the necessary countermeasures.
You also learned about secure coding conventions such as input validation and careful use of the built-in system modules used to run commands. By implementing these strategies, you can bolster the security of your Python applications, thwart potential attacks, and contribute to the overall resilience of your software.
To further reinforce your development process, consider leveraging tools like Snyk. Snyk’s IDE extensions gives you real-time security feedback, scanning your code as you write and suggesting immediate fixes. It's like having a security expert look over your shoulder, ensuring you write safe and resilient code from the beginning.
Start securing your Python apps
Find and fix Python vulnerabilities with Snyk for free.
No credit card required.
Or Sign up with Azure AD Docker ID Bitbucket
By using Snyk, you agree to abide by our policies, including our Terms of Service and Privacy Policy.