Getting started with PHP static analysis in 2024
March 21, 2024
0 mins readPHP is a popular server-side scripting language that is widely used for web development. PHP developers can ship and deploy more high-quality software products by leveraging static analysis tools that help mitigate PHP code errors, security vulnerabilities, and other issues that can impact the quality and security of the application if not addressed early in the development cycle.
What is static analysis?
Static analysis, also known as static code analysis or source code analysis, is a method of debugging by examining source code before a program is run. It's conducted by applying a set of coding rules to a program's source code. The primary objective of static analysis is to catch and rectify the errors in the early phase of code development.
In the context of PHP, static analysis involves examining PHP code without executing it, with the goal of finding issues, confirming coding standards, and understanding the architecture, among other things.
Here's an example of how a static analysis tool would highlight an issue in PHP code:
<?php
function add(int $left, int $right): int {
return $left + $right;
}
echo add("one", "two");
?>
In this example, the static analysis tool would identify that the arguments "one" and "two" are not integers, thus violating the function's parameter type declaration.
Importance of static analysis in PHP development
Static analysis plays a crucial role in PHP development for several reasons. First, it helps to spot PHP code errors and potential issues in the codebase before the code is pushed to production. This is especially important in a language like PHP — which is dynamically typed, making it easy to introduce type-related errors.
Second, static analysis in PHP can ensure that code adheres to a predefined set of coding standards. This is particularly important in large projects or when working in a team, where maintaining a consistent coding style is critical for code readability and maintainability.
Finally, static analysis can help identify complex or 'smelly' code that might need refactoring. By providing metrics on code complexity, it can serve as a guide for improving the overall quality of code.
Benefits of static analysis
Static analysis, particularly for PHP development, helps developers and security teams:
Improve code quality: Static analysis tools can help developers improve the quality of their code by detecting errors, security vulnerabilities, and violations of coding standards. By fixing these issues early in the development cycle, developers can prevent them from becoming larger problems in the future.
Enhance security: From a security perspective, static analysis tools can help identify potential security vulnerabilities in your codebase. They can detect common security issues such as code injection in PHP, cross-site scripting (XSS), and open redirect vulnerabilities.
Detect code errors early: By detecting issues early on, static analysis can save developers time and resources. It can significantly reduce the amount of time spent on debugging and manual code reviews, freeing up developers to focus on more complex tasks. Get started with free security static analysis scanning using Snyk.
Compliance with standards: Static analysis can help ensure that your codebase complies with industry standards for code quality, security, and maintainability. This is particularly important in regulated industries, where compliance with certain standards is often a requirement.
Understanding PERCS 2.0
PERCS 2.0, standing for PHP Enhanced Review Coding Standard, is a significant upgrade to the initial version of PERCS. It is an advanced tool for static analysis of PHP code, enabling developers to adhere to a specific set of coding standards, improve the quality of their code, and strengthen coding conventions.
This version of PERCS provides an array of features, including syntax checking, code style checking, and detection of potential security loopholes. It's a valuable tool for PHP developers aiming to improve the quality of their code and ensure compliance with standard coding conventions.
// Sample PHP code
<?php
$a = 1;
$b = 2;
// PERCS 2.0 can identify if this code adheres to the defined coding standards
$result = $a + $b;
// PERCS 2.0 will provide feedback on potential issues in this code
$result = add('1', 2);
?>
Adhering to coding standards is crucial in software development. They ensure that the code is readable, maintainable, and consistent, making it easier for teams to collaborate and for new developers to understand the codebase. Coding standards also prevent common coding errors and potential security vulnerabilities. By enforcing a standardized coding style, developers can identify and fix issues more efficiently and effectively.
// A non-standard code
<?php
function ADD($a,$b){return $a+$b;}
?>
// A standard code
<?php
function addNumbers($firstNumber, $secondNumber) {
return $firstNumber + $secondNumber;
}
?>
PERCS 2.0 significantly enhances both code quality and adherence to coding conventions. It does this by providing developers with immediate feedback on their code, highlighting areas of non-compliance with coding standards and potential security vulnerabilities.
By using PERCS 2.0, developers can enforce a higher level of code quality, ensuring that their PHP applications are reliable, maintainable, and secure.
Top 3 PHP static analysis tools
In the domain of code security and software development, static analysis is a key factor in maintaining high code quality and identifying potential security vulnerabilities before they become problematic. Let's explore three powerful PHP static analysis tools: PHPStan, Psalm, and Snyk. These tools allow developers to find bugs and security flaws in PHP code, ensuring the application's security compliance.
1. PHPStan
PHPStan is one of the most popular static analyzers for PHP code. It focuses on finding bugs in your code without actually running it. The main benefit of PHPStan is its ability to catch whole classes of bugs even before you write tests for the code.
To install PHPStan, you can use composer, a tool for dependency management in PHP. Here's how:
composer require --dev phpstan/phpstan
Running PHPStan on your PHP code is as simple as executing the command below:
vendor/bin/phpstan analyse src
Here, src
represents the directory containing your PHP code. PHPStan will then output a list of potential issues it found in your code. The output typically includes the file name, line number, and a description of the issue. For example, it may detect and report the following output:
------ ----------------------------------------------------------------
Line src/Example.php
------ ----------------------------------------------------------------
10 Parameter #1 $a of method Example::add() expects int, string given.
------ ----------------------------------------------------------------
2. Psalm
Psalm is another powerful static analysis tool for PHP. It's designed to identify both syntax and logic errors in your code. One of the major benefits of Psalm is its ability to infer types in your PHP code, which can catch type-related bugs before they happen.
You can install Psalm using composer:
composer require --dev vimeo/psalm
To run Psalm on your PHP code, use the following command:
vendor/bin/psalm
Psalm's output will show you a list of issues with your code, similar to PHPStan. However, Psalm also provides a severity level for each issue, which can help prioritize fixes.
Here's a sample output from Psalm:
ERROR: InvalidArgument - src/Example.php:10:10 - Argument 1 of Example::add expects int, string provided
3. Snyk for Code Security in PHP Development
Snyk is a developer-first security tool that helps developers find, understand, and fix vulnerabilities and security issues in their code, open source dependencies, container images, and more. The tool is designed to fit right into your existing workflow. It integrates seamlessly with various IDEs and build tools, so you can easily install the PHPStorm plugin or VS Code extension from Snyk, for free, and start scanning your code for security vulnerabilities.
Snyk identifies insecure code issues by leveraging its extensive vulnerability database and intelligent code analysis capabilities. These capabilities enable the tool to detect a wide variety of potential security threats, including code execution that affected the SuiteCRM project, and also remote code execution in dompdf.
Let's look at an SQL injection example that Snyk can detect:
// Example of SQL Injection Vulnerability
<?php
$conn = new mysqli("127.0.0.1", "user", "MySecretPassword", "db");
function doSqlQuery() {
$query = "SELECT * FROM users WHERE login = '" . $_POST["login"];
return mysqli_query($conn, $query);
}
$target = $_GET["target"];
header("Location: " . $target);
echo $_GET["user"];
In the above PHP code snippet, user inputs are directly concatenated into the SQL query, making it susceptible to SQL injection attacks. Snyk's PHP static code analysis will flag this as a severe security risk. I encourage you to take the Snyk Learn lesson on SQL injections to understand why the above is insecure code and how to prevent this vulnerability.
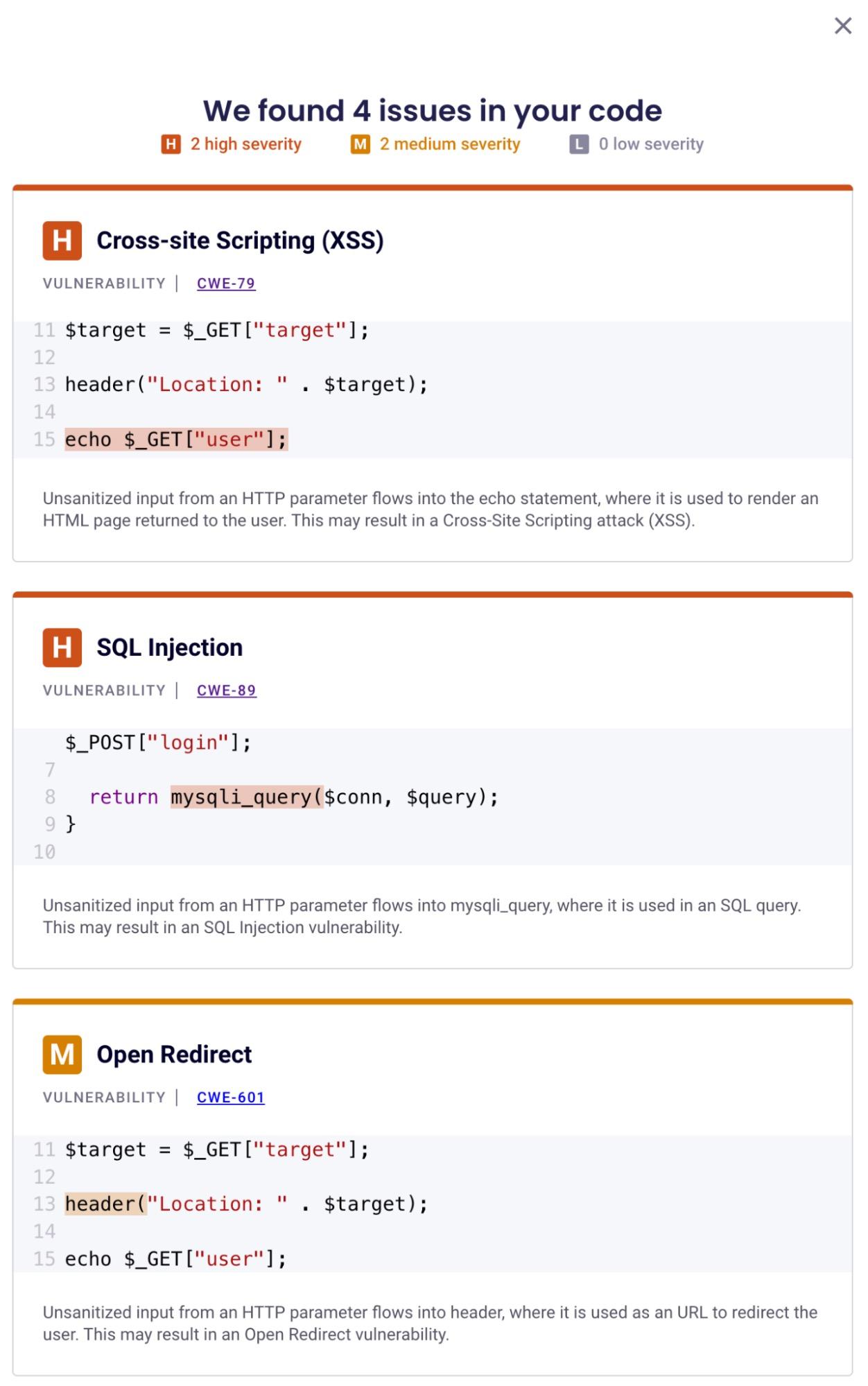
Snyk also helps identify common bugs in PHP code that could lead to security issues. For instance, using a deprecated or unsupported PHP function can introduce vulnerabilities into the application.
Integrating Snyk into PHPStorm and VS Code IDE
Snyk can be easily integrated into popular IDEs like PHPStorm and Visual Studio Code, providing real-time feedback and vulnerability scanning as you code.
After installing the Snyk plugin for PHPStorm, you can run Snyk scans directly from your IDE. Simply right-click on your project in the Project Explorer, select Snyk Scan, and the scan results will be displayed in the Snyk tab in the bottom panel.
Snyk Integration with VS Code
To integrate Snyk with VS Code, you'll need to install the Snyk Vulnerability Scanner extension from the VS Code marketplace. Once installed, Snyk will automatically scan your project for insecure PHP code patterns and vulnerable dependencies in your composer file. You can also rescan a project by opening the Command Palette (Ctrl+Shift+P
), typing Snyk, and selecting the Snyk: Rescan option. The scan results will be displayed in a new Snyk tab in the sidebar.
Integrating PHP static analysis into CI/CD pipelines
With the increasing complexity of software development and the growing need for secure applications, it is more important than ever to integrate static analysis and code style tools into your PHP development workflow. Continuous integration (CI) and continuous deployment (CD) pipelines provide an ideal environment for automating these processes, ensuring that code quality and security are maintained throughout the development lifecycle.
These tools not only help in maintaining code quality and consistency but also act as a first line of defense against potential security threats. They help in identifying and rectifying potential vulnerabilities in the early stages of software development, thereby saving time, effort, and resources in the long run.
# An example of integrating PHPStan into a CI/CD pipeline using GitHub Actions
name: PHPStan
on: [push, pull_request]
jobs:
analysis
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- uses: shivammathur/setup-php@v2
with:
php-version: '7.4'
tools: phpstan
- run: composer install --no-progress
- run: phpstan analyse
In the example above, PHPStan is integrated into a CI/CD pipeline using GitHub Actions. This ensures that static analysis is performed automatically on every push or pull request, catching potential issues early and making code review more efficient.
Benefits of integrating static analysis into CI automation
Integrating static analysis into your CI pipeline can provide numerous benefits:
Early bug detection: Static analysis can help detect potential issues and vulnerabilities early in the development process, reducing the cost and time to fix them.
Improved code quality: By enforcing coding standards and best practices, static analysis can lead to cleaner, more maintainable code.
Enhanced security: Static analysis can help identify security vulnerabilities in your code, leading to more secure applications.
Learning about code style tools
The next topic is about maintaining a consistent code style. Code style tools play a crucial role in enforcing a uniform style across a codebase, making the code readable and maintainable. These tools can also reduce the likelihood of errors and security vulnerabilities by enforcing good coding practices. We can achieve that with dedicated PHP code style tools: PHPCS, StyleCI, and PHP-CS-Fixer.
Why implement a code style tool?
Coding standards are a set of guidelines and best practices for writing code. They help developers to write clean, professional, and error-free code. Following a consistent code style improves code readability, reduces complexity, and promotes code reuse. This is where code style tools come into play. They automatically check the code for style issues and can even fix them in some cases.
In PHP development, these tools are indispensable. They not only help in maintaining a consistent code style but also play a vital role in detecting potential security vulnerabilities. This is essential in today's cyber threat landscape, where the slightest of coding errors can lead to severe security breaches.
PHP_CodeSniffer (PHPCS)
PHP_CodeSniffer (PHPCS) is a set of two PHP scripts. The main phpcs
script tokenizes PHP, JavaScript, and CSS files to detect violations of a defined coding standard and a second phpcbf
script to correct coding standard violations automatically.
You can install PHPCS using composer:
composer global require "squizlabs/php_codesniffer=*"
Once installed, you can run the phpcs
command in your project directory:
phpcs /path/to/your/php/files
PHPCS will output any coding standards violations it finds. For example:
FILE: /path/to/your/php/files/example.php
--------------------------------------------------------------------------------
FOUND 1 ERROR AFFECTING 1 LINE
--------------------------------------------------------------------------------
20 | ERROR | [x] Line indented incorrectly; expected 4 spaces, found 2
--------------------------------------------------------------------------------
PHPCBF CAN FIX THE 1 MARKED SNIFF VIOLATIONS AUTOMATICALLY
--------------------------------------------------------------------------------
You can use the phpcbf
command to automatically fix these issues:
phpcbf /path/to/your/php/files
StyleCI
StyleCI is a PHP coding style continuous integration service. It's a web-based tool that you integrate with your GitHub, GitLab or Bitbucket repositories. It checks every push or pull request to ensure the code adheres to the configured coding style.
To use StyleCI, you need to sign up on their website and link your repositories.
PHP-CS-Fixer
The PHP-CS-Fixer tool by FriendsOfPHP is another tool that not only detects but also fixes coding standards issues in your PHP code.
You can install PHP-CS-Fixer using composer:
composer global require friendsofphp/php-cs-fixer
After installing PHP-CS-Fixer, you can use the php-cs-fixer
command to fix your code:
php-cs-fixer fix /path/to/your/php/files
PHP-CS-Fixer will output the files it fixed:
Fixed all files in 0.123 seconds, 12.000 MB memory used
Conclusion
In this blog post, we have discussed the importance of static analysis and code style tools in the realm of PHP development. We have seen how these tools can improve not only the quality of the codebase, but also the overall security of the application.
Static analysis tools, like PHPStan, Psalm, and Snyk, play a pivotal role in identifying potential issues in your PHP code that could lead to bugs or security vulnerabilities. These tools perform an in-depth analysis of your code without actually executing it, thereby making it possible to catch errors at an early stage.
If you are building PHP applications using containers, I recommend checking out the following resources next:
Get started in capture the flag
Learn how to solve capture the flag challenges by watching our virtual 101 workshop on demand.