10 React security best practices

July 18, 2022
0 mins readEditor's note
The date on this post reflects its latest update. This post was originally published on October 28, 2020.
Looking for the best ways to secure your React app? Then you’ve come to the right place! We’ve created this checklist of React security best practices to help you and your team find and fix security issues in your React applications. We’ll also show you how to automatically test your React code for security-related issues and automatically fix them.
Let’s get to it!
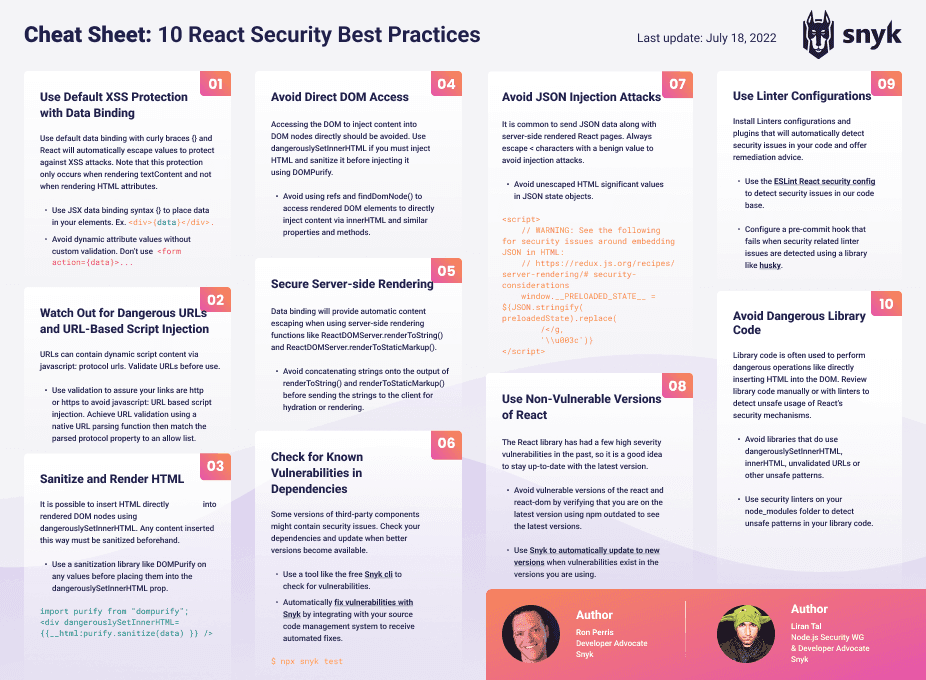
10 React security best practices
Use default XSS protection with data binding
Watch out for dangerous URLs and URL-based script injection
Sanitize and render HTML
Avoid direct DOM access
Secure React server-side rendering
Check for known vulnerabilities in dependencies
Avoid JSON injection attacks
Use non-vulnerable versions of React
Use linter configurations
Avoid dangerous library code
1. Use default React XSS protection with data binding
Is React vulnerable to cross-site scripting (XSS)?
By default, React is built to not be vulnerable, but there are options that developers can enable which could make it vulnerable to cross-site scripting.
How can I prevent React XSS attacks?
Use default data binding with curly braces ({}
) and React will automatically escape values to protect against XSS attacks. Note that this protection only occurs when rendering textContent
and not when rendering HTML attributes.
Use JSX data binding syntax ({}
) to place data in your elements.
Do this:
<div>{data}</div>
Avoid dynamic attribute values without custom validation.
Don’t do this:
<form action={data}>...
2. Watch out for dangerous URLs and URL-based script injection
URLs can contain dynamic script content via javascript:
protocol URLs. Use validation to assure your links are http:
or https:
to avoid javascript:
URL-based script injection. Achieve URL validation using a native URL parsing function then match the parsed protocol property to an allow list.
Do this:
function validateURL(url) {
const parsed = new URL(url)
return ['https:', 'http:'].includes(parsed.protocol)
}
<a href={validateURL(url) ? url : ''}>Click here!</a>
Don’t do this:
<a href={attackerControlled}>Click here!</a>
3. Sanitize and render HTML
It is possible to insert HTML directly into rendered DOM nodes using dangerouslySetInnerHTML
. Any content inserted this way must be sanitized beforehand. Use a sanitization library like dompurify
on any values before placing them into the dangerouslySetInnerHTML
prop.
Use dompurify
when inserting HTML into the DOM.
Do this:
import purify from "dompurify";
<div dangerouslySetInnerHTML={{ __html:purify.sanitize(data) }} />
4. Avoid direct DOM access
Always avoid accessing the DOM to inject content into DOM nodes directly. But if you do have to, use dangerouslySetInnerHTML
to inject HTML and sanitize it before injecting it using dompurify
.
What is react dangerouslySetInnerHTML
?
dangerouslySetInnerHTML
is a property that enables developers to directly insert html content within an HTML element found in a React app.
Do this:
import purify from "dompurify";
<div dangerouslySetInnerHTML={{__html:purify.sanitize(data) }} />
Avoid using refs and findDomNode()
to access rendered DOM elements to directly inject content via innerHTML
and similar properties or methods.
Don’t do this:
this.myRef.current.innerHTML = attackerControlledValue;
5. Secure React server-side rendering
Data binding will provide automatic content escaping when using server-side rendering functions like ReactDOMServer.renderToString()
and ReactDOMServer.renderToStaticMarkup()
.
Avoid concatenating strings onto the output of renderToStaticMarkup()
before sending the strings to the client for hydration.
To avoid XSS, don’t concatenate unsanitized data with the output of renderToStaticMarkup()
.
Do this:
app.get("/", function (req, res) {
return res.send(
ReactDOMServer.renderToStaticMarkup(
React.createElement("h1", null, "Hello World!")
) + otherData
);
});
6. Check for known vulnerabilities in dependencies
Some versions of third-party components might contain JavaScript security issues. Always check your dependencies with a software composition analysis (SCA) tool before adding them to a project, and be sure to update when a newer version becomes available.
Snyk VS Code extension
While developing a React application in VS Code you can use the Snyk extension to alert you of any known vulnerabilities in your project. You’ll find visibility to these alerts via squiggly line warnings directly in your package.json file, the Snyk extensions panel and the VS Code problems panel. This is helpful so that you don’t have to switch context out of your development environment to learn of these vulnerabilities — it’s all within your scope.
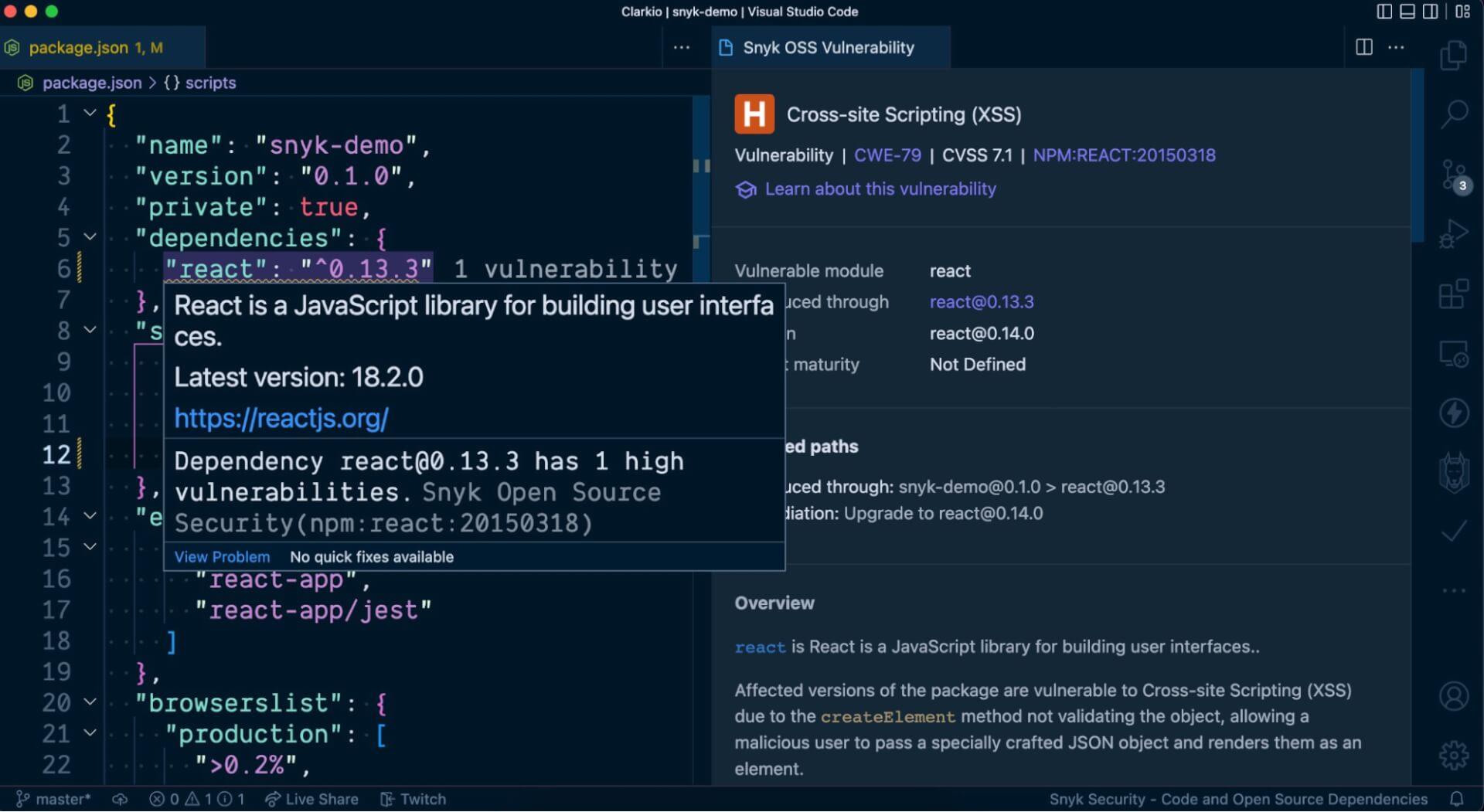
Snyk app (https://app.snyk.io)
Opening a PR to fix a vulnerability in React is as simple as three clicks. First, identify the vulnerability you wish to address in your app using the Snyk web app. Then click Fix this vulnerability (or Fix these vulnerabilities if there are multiple). Learn more about the Snyk product here.
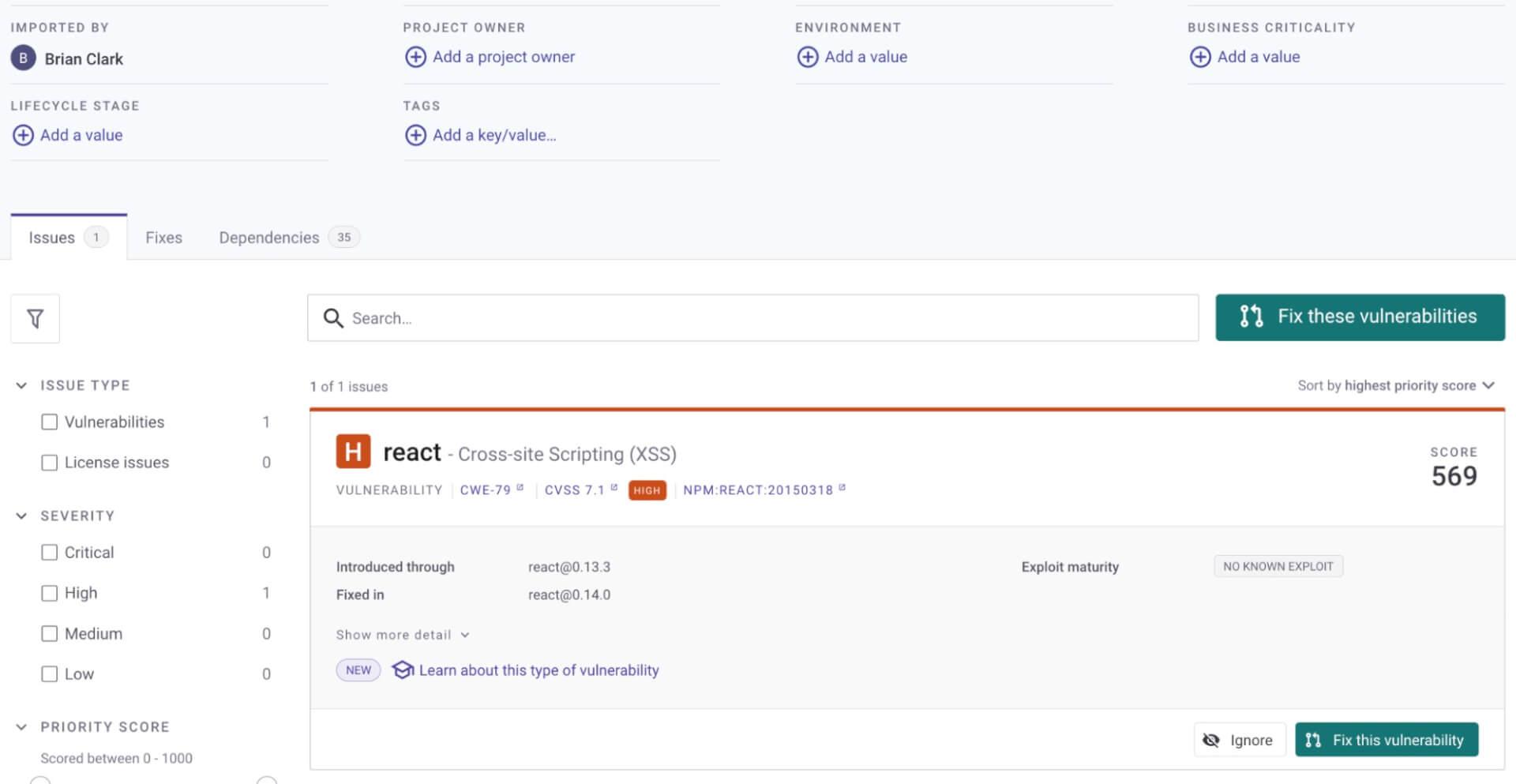
You’ll then be prompted to confirm you wish to open a pull request (PR) to fix the vulnerability using Snyk.
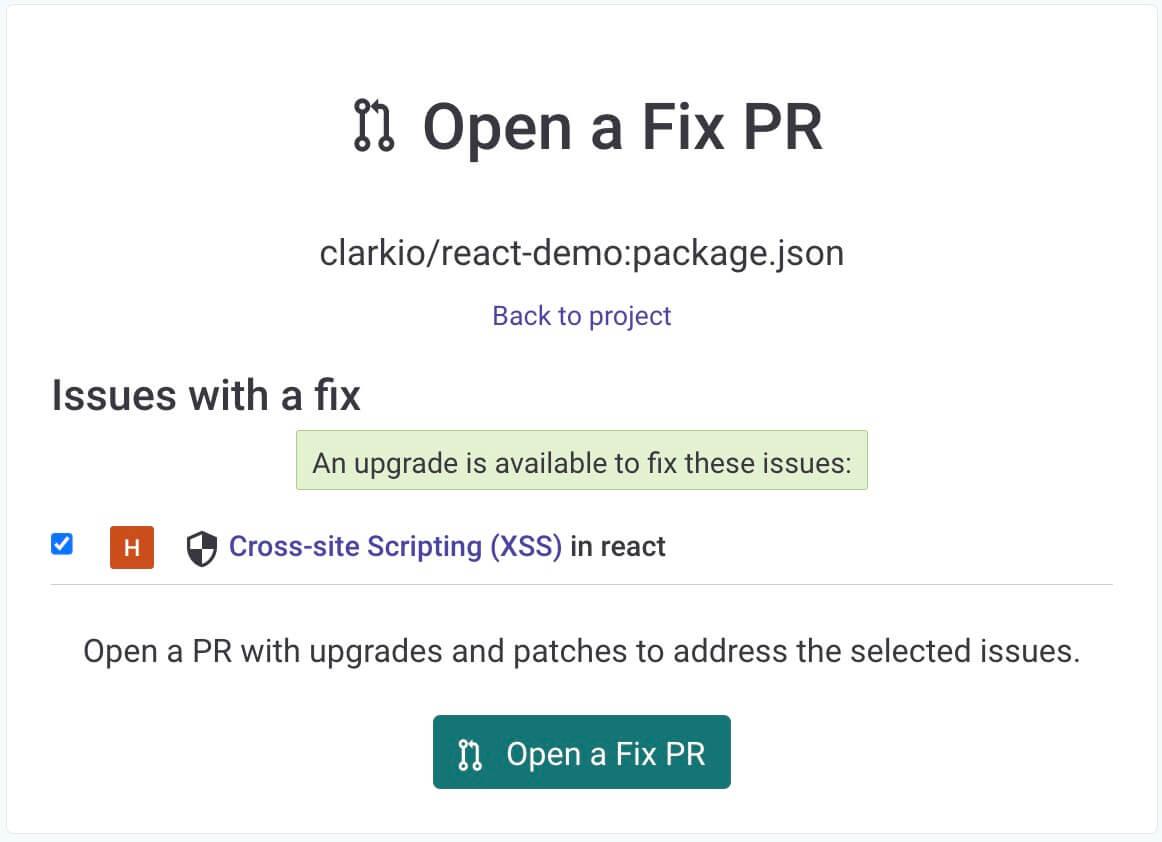
After confirming you’ll be brought to the PR that was opened by Snyk so you can review and test that your app still works as expected with the changes requested before merging them.
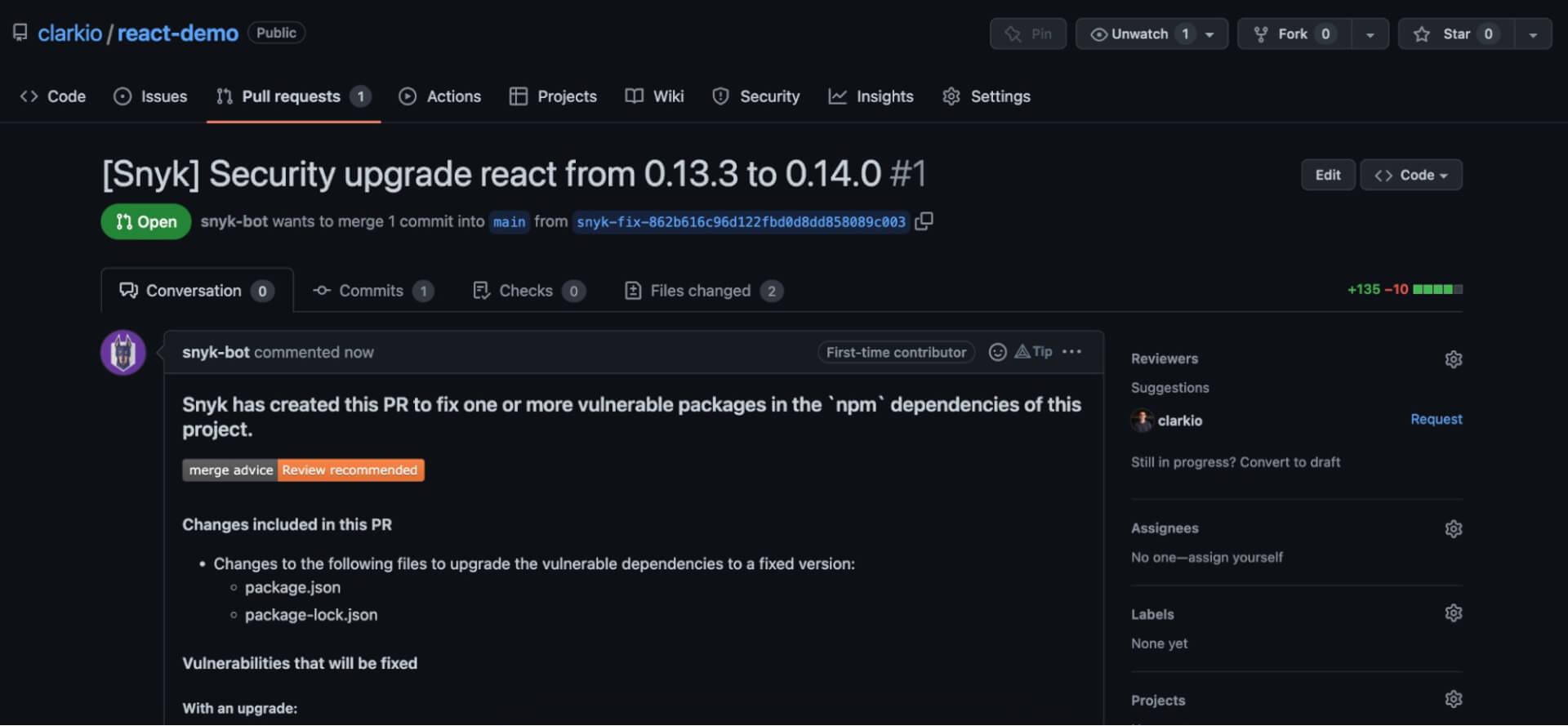
Snyk CLI
You can also use the free Snyk CLI to check for vulnerabilities. Automatically fix vulnerabilities with Snykby integrating with your source code management system to receive automated fixes:
$ npx snyk test
Get started in capture the flag
Learn how to solve capture the flag challenges by watching our virtual 101 workshop on demand.
7. Avoid JSON injection attacks
It is common to send JSON data along with server-side rendered React pages. Always escape <
characters with a benign value to avoid injection attacks.
Always escape HTML significant values from JSON with benign equivalent characters.
Do this:
window.__PRELOADED_STATE__ = ${JSON.stringify(preloadedState).replace( /</g, '\\u003c')}
8. Use non-vulnerable versions of React
The React library has had a few high severity vulnerabilities in the past, so it is a good idea to stay up to date with the latest version.
Avoid vulnerable versions of the react
and react-dom
by verifying that you are on the latest version using npm outdated to see the latest versions.
9. Use linter configurations
Install linter configurations and plugins that will automatically detect security issues in your code and offer remediation advice.
Use the ESLint React security config to detect security issues in our code base.
Configure a pre-commit hook that fails when security-related linter issues are detected using a library like husky.
Use Snyk to automatically update to new versions when vulnerabilities exist in the versions you are using.
10. Avoid dangerous library code
Library code is often used to perform dangerous operations like directly inserting HTML into the DOM. Review library code manually or with linters to detect unsafe usage of React’s security mechanisms.
Avoid libraries that do use dangerouslySetInnerHTML
, innerHTML
, unvalidated URLs or other unsafe patterns. Use security linters on your node_modules
folder to detect unsafe patterns in your library code.
Download the React security cheat sheet
Download this handy PDF and use it as a checklist when verifying the security of your React application.