In diesem Abschnitt
Simple Doesn't Always Mean Secure: Avoid this Golang XSS Pattern
26. Februar 2025
4 Min. LesezeitCross-site scripting (XSS) attacks are a typical web vulnerability that allows attackers to inject malicious scripts into web pages viewed by other users. You’d think that keeping things simple would lead to more secure code. No third-party dependencies, less abstractions. It seems ideal, but is it?
This article will demonstrate a simple web server, the Golang program, and emphasize why simple doesn’t always mean secure.
About Cross-site Scripting Attacks
Cross-site Scripting (XSS) is scripts that often steal user data, hijack sessions, or deface websites. While Golang offers robust web development tools, it is not immune to XSS vulnerabilities if proper precautions aren't taken.
Example of Cross-site Scripting in Golang
A frequent source of XSS vulnerabilities arises from directly embedding user-supplied input into HTML output without proper sanitization or other security controls. For reference, effective security control isn’t always sanitization but rather output encoding.
An example of a Go application is one that receives data from a user via a form field or URL parameter (such as a query string) and then directly inserts this data into an HTML page as part of the HTTP response. An attacker could inject malicious JavaScript code (therefore, the name Cross-site Scripting). When another user views the page, their browser will execute the injected script, potentially compromising their data or session.
In Golang HTTP servers, a common mistake is using fmt.Fprint
or similar functions to write user-provided data directly into the HTTP response. Consider the following code snippet:
http.HandleFunc("/greet", func(w http.ResponseWriter, r *http.Request) {
name := r.URL.Query().Get("name")
response := fmt.Sprintf("<html><body><h1>Hello, %s!</h1></body></html>", name)
fmt.Fprint(w, response)
})
If a user sets the name
query string to an input such as <script>alert('XSS!')</script>
, this code will inject the JavaScript alert()
code into the page, demonstrating a basic XSS attack. The fmt.Sprintf
function doesn't automatically escape HTML entities, leaving the application vulnerable.
That Golang code snippet is part of an open source security challenge. Snyk imported this code repository and was able to detect the existence of unsanitized input from the request URL and understand how it flows into the insecure fmt.Fprint
function call that renders the HTML template string. A full source-code and code path analysis by Snyk’s SAST demonstrates the findings in more detail:
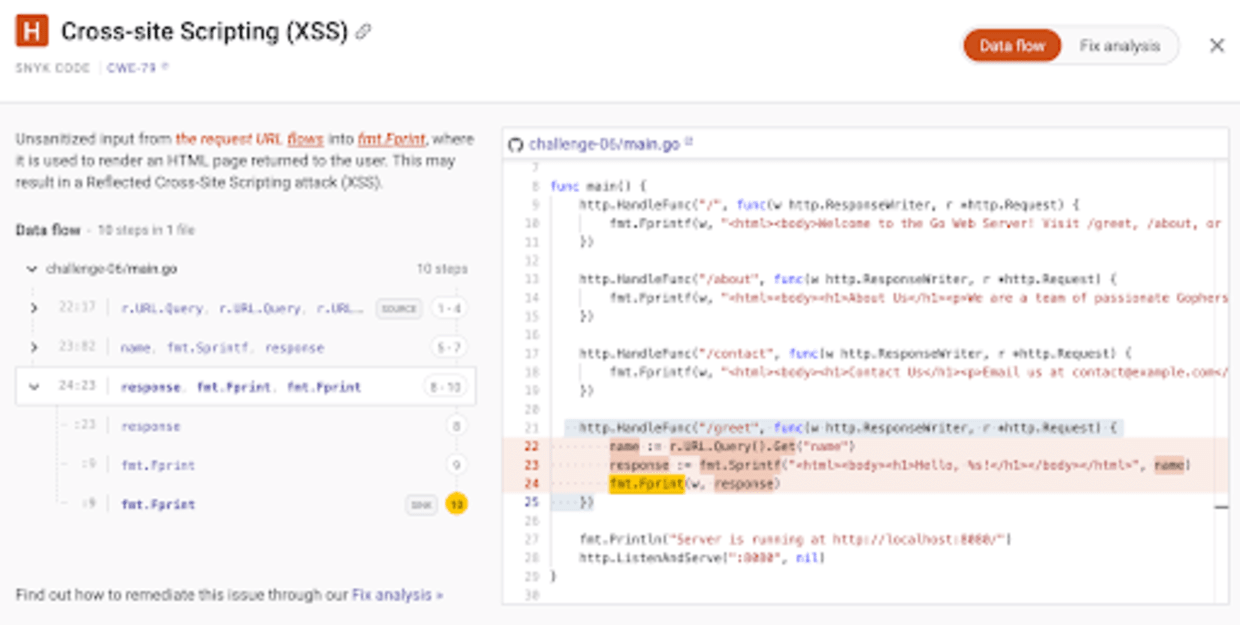
How to fix XSS in Golang
The vulnerable Golang example above emphasizes the need for proper escaping or sanitization of user inputs before they are included in HTML responses.
Escaping potentially dangerous HTML code means that the application’s code or the third-party dependency relied upon would need to encode specific user input to avoid being interpreted natively as HTML DOM elements. For example, frontend frameworks such as React or Vue translate the character <
to its HTML entity equivalent, which is <
.
Does it mean that you should always rely on third-party dependencies? Well, no, but if you actively monitor and scan them for security vulnerabilities and keep them under proper maintenance with automated updates, it is easier to control the security risk. There’s then also the concern of misusing the library in a way that would still introduce a vulnerability.
What about secure code issues in Golang? There’s a broad Snyk support for Go regarding Go code, Go dependencies, and package managers.
Kostenloser Online-Code-Check
Ihr Code. Unser Commitment. Hier machen Sie Ihre Commits sicher.