Commit 8e3c406
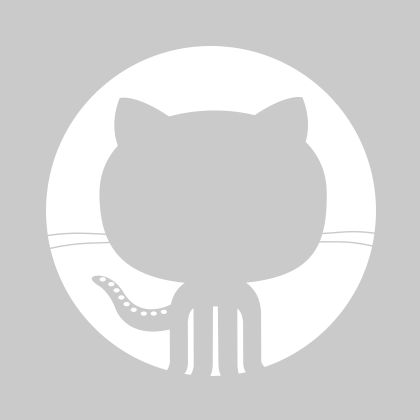
Eunjae Lee
1 parent 97c5ce4 commit 8e3c406
File tree
3 files changed
+84
-11
lines changed- src/lib
- __tests__
- test/mock
3 files changed
+84
-11
lines changedOriginal file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
154 | 154 |
| |
155 | 155 |
| |
156 | 156 |
| |
157 |
| - | |
| 157 | + | |
| 158 | + | |
| 159 | + | |
| 160 | + | |
158 | 161 |
| |
159 | 162 |
| |
160 | 163 |
| |
| |||
263 | 266 |
| |
264 | 267 |
| |
265 | 268 |
| |
266 |
| - | |
267 |
| - | |
268 |
| - | |
269 | 269 |
| |
270 | 270 |
| |
271 | 271 |
| |
272 | 272 |
| |
273 |
| - | |
| 273 | + | |
| 274 | + | |
| 275 | + | |
| 276 | + | |
274 | 277 |
| |
275 | 278 |
| |
276 | 279 |
| |
| |||
285 | 288 |
| |
286 | 289 |
| |
287 | 290 |
| |
| 291 | + | |
| 292 | + | |
| 293 | + | |
| 294 | + | |
| 295 | + | |
| 296 | + | |
| 297 | + | |
| 298 | + | |
| 299 | + | |
| 300 | + | |
| 301 | + | |
| 302 | + | |
| 303 | + | |
| 304 | + | |
| 305 | + | |
288 | 306 |
| |
289 | 307 |
| |
290 | 308 |
| |
| |||
456 | 474 |
| |
457 | 475 |
| |
458 | 476 |
| |
459 |
| - | |
460 |
| - | |
| 477 | + | |
| 478 | + | |
461 | 479 |
| |
462 | 480 |
| |
463 | 481 |
| |
| |||
497 | 515 |
| |
498 | 516 |
| |
499 | 517 |
| |
500 |
| - | |
501 |
| - | |
| 518 | + | |
| 519 | + | |
502 | 520 |
| |
503 | 521 |
| |
504 | 522 |
| |
| |||
576 | 594 |
| |
577 | 595 |
| |
578 | 596 |
| |
579 |
| - | |
580 |
| - | |
| 597 | + | |
| 598 | + | |
581 | 599 |
| |
582 | 600 |
| |
583 | 601 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1576 | 1576 |
| |
1577 | 1577 |
| |
1578 | 1578 |
| |
| 1579 | + | |
| 1580 | + | |
| 1581 | + | |
| 1582 | + | |
| 1583 | + | |
| 1584 | + | |
| 1585 | + | |
| 1586 | + | |
| 1587 | + | |
| 1588 | + | |
| 1589 | + | |
| 1590 | + | |
| 1591 | + | |
| 1592 | + | |
| 1593 | + | |
| 1594 | + | |
| 1595 | + | |
| 1596 | + | |
| 1597 | + | |
| 1598 | + | |
| 1599 | + | |
| 1600 | + | |
| 1601 | + | |
| 1602 | + | |
| 1603 | + | |
| 1604 | + | |
| 1605 | + | |
| 1606 | + | |
| 1607 | + | |
| 1608 | + | |
| 1609 | + | |
| 1610 | + | |
| 1611 | + | |
| 1612 | + | |
| 1613 | + | |
| 1614 | + | |
| 1615 | + | |
| 1616 | + | |
| 1617 | + | |
| 1618 | + | |
| 1619 | + | |
| 1620 | + | |
| 1621 | + | |
| 1622 | + | |
| 1623 | + | |
| 1624 | + | |
| 1625 | + | |
| 1626 | + | |
| 1627 | + | |
| 1628 | + | |
| 1629 | + | |
| 1630 | + | |
| 1631 | + | |
| 1632 | + | |
1579 | 1633 |
| |
1580 | 1634 |
| |
1581 | 1635 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
51 | 51 |
| |
52 | 52 |
| |
53 | 53 |
| |
| 54 | + | |
54 | 55 |
| |
55 | 56 |
| |
56 | 57 |
| |
|
0 commit comments